What is the purpose of the View component in React Native?
The View component is key for building a user interface (UI) in React Native. It acts as a container for layout, style, touch handling, and accessibility. It directly maps to native views on platforms like iOS, the web, or Android.
React Native lets developers build Android and iOS apps with JavaScript. This is different from traditional development, which often uses Kotlin, Java, Swift, or Objective-C. React Native makes it easier to create apps that look and feel like native ones on both platforms.
React Native offers many Core Components, like , , , , and . These components work well on Android, iOS, and the web. They ensure a smooth user experience across platforms. The community also provides custom Native Components, listed in the Native Directory, to enhance app functionality.
Introduction to React Native
React Native is an open-source framework for building mobile apps. It uses the React JavaScript library and native capabilities. This lets developers from different backgrounds create fast, high-quality apps that work well with the operating system.
The framework has detailed guides for all experience levels. It helps you set up a development environment and move from web to mobile. It also has the Snack Player for interactive project development and previews on Android and iOS.
React Native stands out because it uses native components for a better user experience. It focuses on the strengths of mobile platforms. This makes apps more authentic and efficient.
If you already know React, learning React Native is easy. It uses familiar concepts like JSX, components, state, and props. Plus, it supports the latest JavaScript features.
React Native lets you write markup in JavaScript with JSX. This makes development easier and leads to a more consistent UI.
React Native also lets you customize components a lot. You can change how they look and work. This makes your app more efficient and scalable.
Handling state in React Native is the same as in React web development. This makes it easy for developers to adapt.
Understanding the View Component
The View component is key for making user interfaces in React Native. It’s a container that doesn’t scroll and supports layout with flexbox. It also handles styling, touch, and accessibility.
The View component matches the native view on each platform. For example, it’s UIView on iOS and android.view on Android.
Fundamental UI Building Block
The View component is used inside other views and can hold many children. It’s the basic way to organize content in a React Native app. Developers use it to build complex interfaces from smaller parts.
Flexbox Layout Support
React Native supports flexbox layout. This lets developers control how elements are sized, placed, and aligned in a View. It helps make interfaces that work well on all screen sizes and orientations.
Learning about the View component and its layout options is crucial. It helps React Native developers make apps that look good and work well on both iOS and Android.
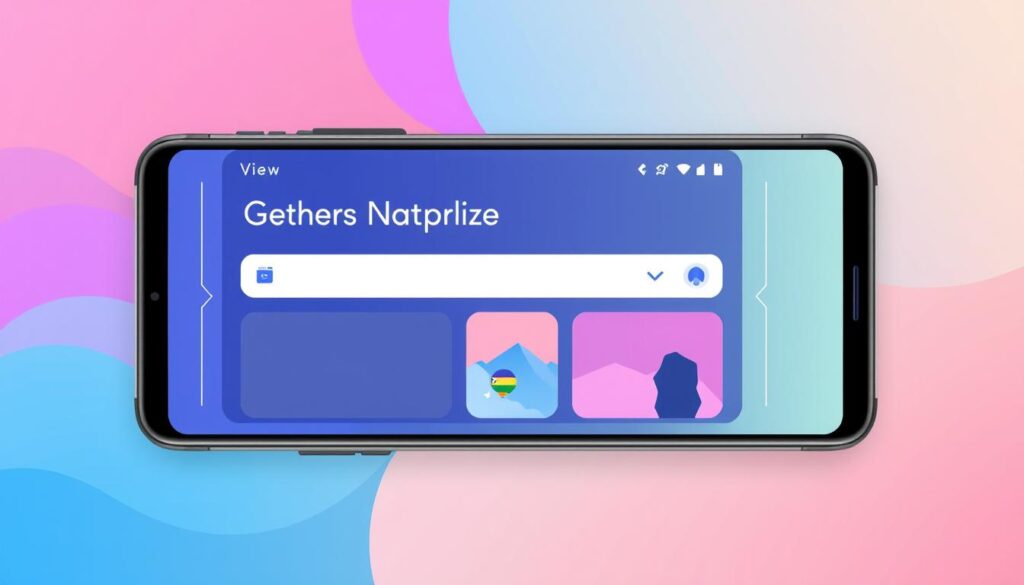
Develop applications using React Native
React Native has quickly become a top choice for making mobile apps. Famous apps like Facebook and Instagram use it. It lets developers make fast, high-quality apps for both Android and iOS.
There are two ways to make React Native apps: using React Native CLI or Expo. Expo is great for simple apps or quick tasks. It’s perfect for MVPs or PoCs when you need to work fast and save money.
React Native has a cool feature called hot reloading. It helps developers test ideas quickly without spending a lot. When making MVPs or PoCs, remember to follow best practices like using TypeScript and focusing on performance.
But, React Native has its challenges. You might need to work on custom UIs and test your app on different platforms. Luckily, it has a big community that helps solve these problems.
It’s easy to find React Native developers to join your team. This is because of the big community and lots of resources. If you already use React or need JavaScript help later, React Native is a good pick.
MobiDev knows how to make React Native apps for many industries. They use a dedicated team approach. With React Native, you can make apps for both Android and iOS with just one codebase. It’s a strong tool for cross-platform mobile development.
Key Features of the View Component
The View component in React Native is key for making mobile app interfaces. It’s great at handling touch actions like tapping, swiping, and dragging. This makes it easy for developers to add user interactions to their apps.
Touch Handling Capabilities
The View component has many touch handling features. These help make apps more interactive and easy to use. Here are some of its main features:
- Tap detection: It can recognize and handle tap gestures. This lets users interact with different parts of the app.
- Swipe recognition: Developers can add swipe actions. This is useful for scrolling or moving through content.
- Drag and drop: It supports drag and drop. This lets users move and arrange elements in the app.
Accessibility Controls
The View component also has strong accessibility controls. This makes sure apps are open to everyone, not just those without disabilities. Here are some of its accessibility features:
- Accessibility labels: Developers can add labels to View components. This helps users with screen readers or other tools understand the app better.
- Accessibility traits: It lets developers set traits like “button” or “header.” This tells users with disabilities what each element does.
- Accessibility actions: Developers can create custom actions. This lets users interact with View components in unique ways, like double-tapping or long-pressing.
Using the View component’s touch handling and accessibility features, developers can make apps that are both interactive and inclusive. This way, apps can meet the needs of a wide range of users.
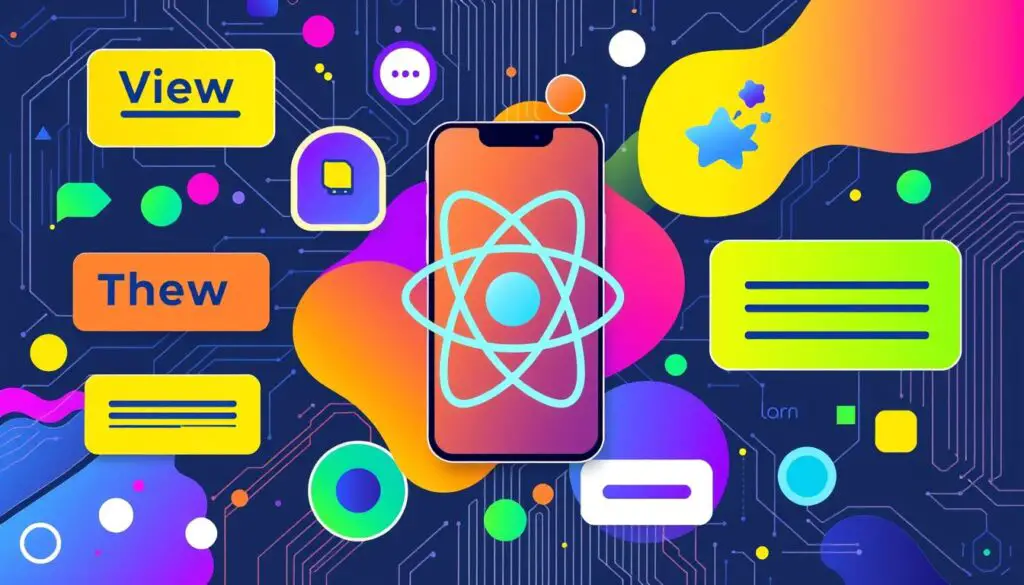
Styling the View Component
In React Native, the View component is key for building user interfaces. It can be styled using the StyleSheet API. This makes it easy to create styles that look good everywhere.
The StyleSheet module in React Native helps manage styles well. You can keep all your styles in one place. This makes it simple to change how your app looks. You can use properties like borderRadius for rounded corners and flex for layouts that adjust.
Using the StyleSheet module, developers can make React Native apps that look great. They can customize the look of their apps a lot. This is important for making mobile apps that look good and work well.
Metric | Value |
---|---|
Number of design sections subdivided for implementation | 4 |
Total number of lines in the initial screen before clean up | 3 |
Total number of lines in the screen after simplification | 4 |
Number of Flexbox properties explained | 3 (flexDirection, justifyContent, alignItems) |
Total number of sections in the design to implement | 4 |
Total number of dots to be displayed across the top | 3 (One pink, two grey) |
Total number of properties needed to create the Dot component | 2 states (active and inactive) |
Nested Views and Children Components
The View component in React Native is powerful. It lets developers nest views to build complex interfaces. Views can hold many child components, like Text, Image, or custom ones. This feature is key for making detailed layouts and interactions.
Managing nested views and children is crucial for React Native apps. It keeps code organized and running smoothly. If not done right, it can make code hard to manage and slow.
The Flexbox layout system is essential for nested views. It helps create designs that adjust well on different screens. With Flexbox, developers can easily arrange views and their children, making the app look great.
React Native has many tools to help with nested views. The React Dev Tools extension is one. It helps find and fix problems with views and their children, making apps better and easier to keep up.
Metric | Value |
---|---|
React Native usage among top 500 apps | 14.85% of all applications downloaded |
React Native UI library growth | From 232,168 results in 2022 to 355,832 results in 2023 |
React Native preference among developers | 40.58% of respondents in the 2023 Stack Overflow Annual Developer Survey |
In summary, the View component is vital for React Native. It helps build complex, attractive interfaces. With Flexbox and tools, developers can manage views well, making apps efficient and easy to maintain.
Performance Considerations
When making apps with React Native, it’s key to focus on performance. One way to do this is by removing clipped subviews. You can do this by setting the removeClippedSubviews
prop on the View
component.
The removeClippedSubviews
prop tells React Native to remove views that are clipped from the native hierarchy. This makes your app run smoother, especially when there are many off-screen views. By removing these views, React Native does less work, making your app more efficient and responsive.
Removing Clipped Subviews
To use this technique, set the removeClippedSubviews
prop on the View
component like this:
<View removeClippedSubviews>
{/* Your subviews */}
</View>
This is really helpful when you have lots of views that are off-screen, like in a scrollable list. By removing these views, React Native does less work, making your app faster and more responsive.
But, remember to use the removeClippedSubviews
prop wisely. It can cause problems with animations or interactions involving clipped views. So, test it well to make sure it works right for your app and doesn’t cause any issues.
Conclusion
The React Native framework has changed the game in mobile app development. Its View component lets developers create apps that work well on both iOS and Android. This makes apps fast and smooth.
The View component is very flexible and customizable. It supports Flexbox layout and touch handling. It also has accessibility controls and lets developers nest views. This makes it easy to create apps that are both fun and easy to use.
Big companies like Facebook, Instagram, and Airbnb use React Native. This shows how strong and reliable the platform is. As React Native grows, developers can make their work easier and faster.
By using the View component well, developers can make amazing apps. These apps will grab users’ attention and help businesses grow. React Native is a powerful tool for creating top-notch mobile apps.