What is the Navigator widget and how is navigation handled in Flutter?
The Navigator widget is key in Flutter’s UI toolkit. It makes moving between screens easy. Each screen is like a “plate” in a stack.
Developers can add new screens or go back to the last one. This makes navigation simple and efficient.
The Navigator keeps track of the current screen. It’s always at the top of the stack. This makes moving between screens smooth.
It’s important for developers to know how to use the Navigator. It helps in creating apps that work well on different platforms. The Navigator’s methods, like push()
and pop()
, are vital for a good user experience.
Introduction to Navigation in Flutter
Navigation is key in app design, affecting how users use the app. Flutter makes it easy with its routing system. This system helps manage different app pages, making it better for users and developers.
Understanding the Importance of Navigation in App Development
Good navigation makes apps easy to use. It lets users move around the app smoothly. Flutter’s navigation system uses Routes and Navigators to manage this.
Overview of Flutter’s Navigation System
Knowing how to use routes is vital for smooth app navigation. Flutter offers tools like stack-based routing and named routing. These help make apps that are easy and fun to use.
The Navigator class is key for managing routes in Flutter. It uses a stack to handle screen changes. This makes navigation simple and efficient.
Navigation Technique | Description |
---|---|
Stack Routing (Basic Routing) | The standard approach to navigation in Flutter, where routes are managed using a stack-based system. |
Named Routing | A more organized way to manage navigation in larger applications, where routes are referenced by predefined string identifiers. |
Flutter also has many routing packages. These include Auto Route, Go Router, and flutter_modular. They offer features like typed routing and modular setup.
The Route and Navigator Widgets
In Flutter, the Route widget is key for navigation. It has the basic properties and methods for screen transitions. It’s a base class that gets extended for different transition styles and platform needs.
The Navigator widget handles the navigation stack. It makes it easy to move between screens and manage back navigation. This way, the current screen is always at the top.
Role of the Route Widget in Navigation
The Route widget in Flutter is a base class for navigation. It’s used to create different Route classes like MaterialPageRoute, CupertinoPageRoute, and PageRouteBuilder. Each has its own features and uses.
Functionalities of the Navigator Widget
The Navigator widget manages the navigation stack in Flutter. It uses a stack to handle screen transitions. Developers can add new routes or go back to the previous one.
This stack-based approach makes navigation simple. It ensures the current screen is always at the top. This simplifies moving between screens and handling back navigation.
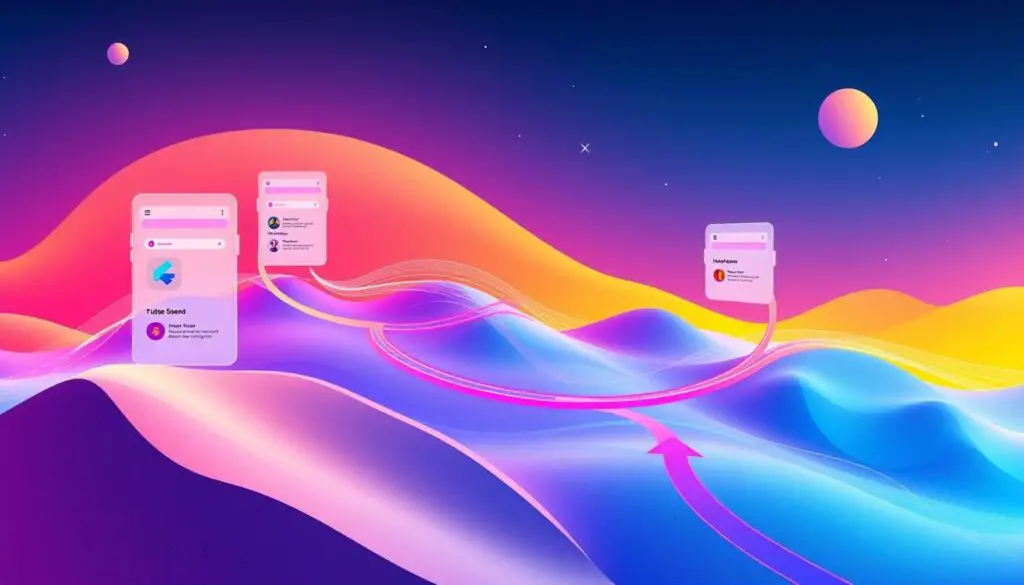
Types of Routes in Flutter
In Flutter UI toolkit, developers can use different types of routes. These help in making apps responsive and use Flutter widgets. The most popular routes in Google Flutter are MaterialPageRoute, CupertinoPageRoute, and PageRouteBuilder.
MaterialPageRoute
MaterialPageRoute uses transitions that follow Material Design rules. It combines fade and scale transitions for a smooth user experience. These transitions are polished and don’t need custom animations.
MaterialPageRoute is great for Android apps. It makes the app feel native to Android users.
CupertinoPageRoute
CupertinoPageRoute is for apps that follow Cupertino design. This design is Apple’s way to make apps elegant and easy to use. It makes sure the app’s navigation is like other iOS apps.
It uses transitions that are similar to iOS’s. For example, it slides pages in from the right and out from the left.
PageRouteBuilder
PageRouteBuilder lets developers create their own route transitions. It’s different from MaterialPageRoute and CupertinoPageRoute because it doesn’t have set transitions. Instead, it lets you control the animations.
With pageBuilder and transitionsBuilder, you can make complex and beautiful transitions. This makes your app’s navigation stand out.
App development using Flutter
Flutter is a mobile app development framework made by Google. It’s known for creating fast, high-quality apps for iOS, Android, and the web. Developers like it because it lets them build apps for many platforms with just one codebase. This saves time and money.
Advantages of Using Flutter for App Development
Flutter is great for app performance. Its graphics engine, Skia, makes animations smooth, even on older devices. This shows Flutter’s focus on fast apps. Plus, its hot reload feature lets developers see changes right away, speeding up app creation.
Flutter also has many pre-designed widgets. These widgets help developers make common app parts quickly. This makes app development faster and more efficient.
Flutter’s Cross-Platform Capabilities
Flutter is known for making cross-platform applications. These apps work on both Android and iOS. Flutter’s code is in Dart, a modern language. It runs directly on devices, making apps fast and smooth.
While React Native has a bigger community, Flutter’s Dart and reactive UI model are unique. They focus on making apps dynamic and engaging. This improves user experience and keeps users interested.
Navigation Stack and Route Operations
In App development using Flutter, the Navigator widget is key. It manages the navigation stack and controls screen flow in apps. The Navigator keeps a stack of Route objects, showing the user’s screen history. This makes handling screen changes and back navigation easier.
Developers use the Navigator to manage the stack and control app flow. They can perform various operations, such as:
- Navigator.push() – Adds a new route to the top, taking the user to a new screen.
- Navigator.pop() – Removes the top route, taking the user back to the previous screen.
- Navigator.pushNamed() – Navigates to a named route, making navigation more flexible and reusable in Google Flutter.
Knowing how to use the Navigator’s stack is crucial for smooth navigation in Flutter widgets. Mastering navigation stack management ensures a seamless user experience. This makes the Flutter performance stand out, offering a user-friendly interface.
Operation | Description | Usage Rate |
---|---|---|
Navigator.push() | Adds a new route to the top of the stack, navigating to the corresponding screen. | High |
Navigator.pop() | Removes the top route from the stack, navigating back to the previous screen. | High |
Navigator.pushNamed() | Navigates to a named route, allowing for more flexible and reusable navigation. | Moderate |
Custom Route Objects | Creating custom Route objects for on-the-fly route creation and management. | Low |
By understanding and mastering the navigation stack and route operations in Flutter, developers can create seamless and intuitive navigation experiences. This enhances the overall user experience of their mobile app development projects.
Named Routes and Deep Linking
Flutter makes it easy to set up named routes. This means developers can move between screens by name, not just by route. It’s great for big mobile app development projects with lots of screens. By using named routes in MaterialApp or CupertinoApp, navigating becomes simpler and cleaner.
Configuring Named Routes in Flutter
To set up named routes in Flutter, add routes to the MaterialApp or CupertinoApp widget. Each route has a unique name for easy navigation. This makes app App development using Flutter more organized and easier to manage.
Limitations of Named Routes
- Named routes handle deep links but can’t be customized. When a new deep link comes in, Flutter just adds it to the Navigator, no matter where you are.
- Also, Flutter doesn’t work with the browser’s forward button for named routes. So, named routes aren’t the best for apps with complex navigation.
Flutter’s Router widget helps with these issues. It gives more control over app navigation and supports deep linking and browser integration. With the Router, developers can make apps with better navigation for their Google Flutter projects.
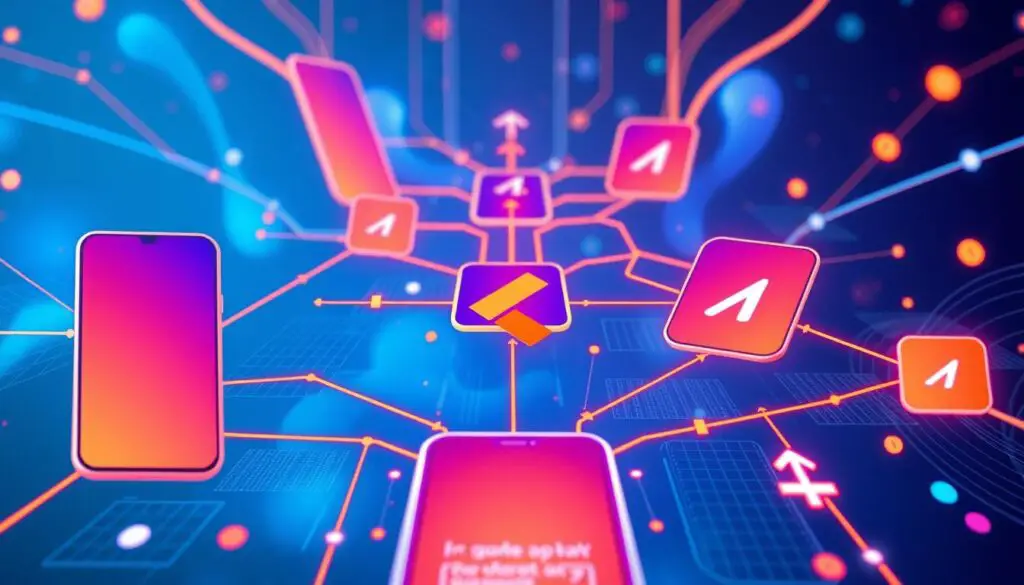
Deep linking in Flutter lets users jump straight to specific content in apps. It boosts user engagement and helps with marketing. Flutter supports deep linking on iOS, Android, and web browsers, making it smooth for users and developers.
Advanced Navigation with the Router
For Flutter apps with complex navigation, using a routing package like go_router is key. These packages offer a more advanced way to navigate. They help developers manage the Navigator based on the route path when a new deep link is received. This ensures a consistent user experience.
Apps with the Router class work well with the browser’s back and forward buttons. When you use the Router to navigate, a new entry is added to the browser’s history. This means pressing the back button takes you to the previous page shown by the Router. It makes navigation smooth and predictable, just like in web apps.
Web Support and Browser Integration
The Router in Flutter makes web apps feel natural. It integrates well with the browser’s back and forward buttons. This ensures a familiar experience for users of web-based Flutter apps.
As Flutter grows, we can look forward to even better navigation features. These updates will make the platform even more powerful for creating top-notch mobile and web apps.
Conclusion
In this article, we’ve looked at how to navigate in App development using Flutter. We’ve seen how the Navigator widget and different routes help make apps easy to use. Whether it’s a simple app or a complex one, knowing how to navigate is key.
The Dart programming language makes Flutter’s UI toolkit strong. It lets apps move smoothly between screens. Flutter’s design makes sure apps look good on all devices.
Google Flutter is getting better and more popular. It focuses on making apps fast and feature-rich. With Flutter’s help, developers can make apps that are easy to use and fun to interact with.