What is Jetpack Compose, and how does it work with Kotlin for UI development?
Jetpack Compose is a modern toolkit for building native Android user interfaces (UI). It makes UI development simpler and faster. It uses Kotlin, a powerful programming language, to help developers create UIs more efficiently.
With Jetpack Compose, developers can write less code and design UIs more intuitively. It uses Kotlin’s features like default arguments and lambdas. This makes the development process smoother and more straightforward.
At the heart of Jetpack Compose is the Kotlin compiler plugin. It turns composable functions into UI elements for Android devices. This means developers can write their UI components in Kotlin, not just XML layouts.
Jetpack Compose works well with Kotlin’s strong features like coroutines and Room database. It also supports dependency injection and Android Architecture Components. These tools help developers build strong, scalable, and easy-to-maintain Android apps.
As Android development keeps changing, Jetpack Compose and Kotlin are becoming key players. They change how developers create UIs and build Android apps. By using this modern toolkit, developers can make their apps more efficient, creative, and high-performing. This leads to better user experiences on the Google Play Store.
Introduction to Jetpack Compose
Jetpack Compose is a modern UI toolkit for Android app development using Kotlin. It helps developers create beautiful user interfaces quickly. It uses a declarative approach to UI building.
Kotlin programming with Jetpack Compose makes UI development faster. It lets developers write UI components in Kotlin code instead of XML. This uses Kotlin’s features like default arguments and lambdas.
Jetpack Compose is a modern toolkit for building native Android UI
Jetpack Compose simplifies building visually appealing and responsive user interfaces for Android app development using Kotlin. It uses Kotlin’s concise syntax and null safety. It also uses extension functions and coroutines for asynchronous programming.
Simplifies and accelerates UI development on Android with less code, powerful tools, and intuitive Kotlin APIs
Jetpack Compose uses composable functions as lightweight building blocks for UI components. These functions, marked with the @Composable annotation, help create complex user interfaces. It also supports managing UI state using state variables and state hoisting techniques.
Jetpack Compose includes Material Design components like buttons and text fields. These components follow Google’s design guidelines. This ensures a consistent and visually appealing user experience across Android app development using Kotlin.
With Android Studio‘s powerful debugging tools and automated testing, developers can quickly fix issues. This optimizes the performance and quality of their Kotlin programming apps.
Feature | Benefit |
---|---|
Composable functions | Lightweight and reusable building blocks for defining UI components |
State management | Built-in support for managing UI state using state variables and state hoisting |
Material Design components | Consistent and visually appealing user experience across Android apps |
Debugging and testing tools | Powerful debugging and automated testing capabilities in Android Studio |
Building UI Components with Declarative Functions
Creating user-friendly interfaces is key in Android App Development Using Kotlin. Jetpack Compose uses Composable functions to define UI components. These functions are annotated with @Composable. They tell the Kotlin compiler how to create the UI elements shown on the screen.
The way Jetpack Compose works is unique. It focuses on what the UI should look like, not how to make it. This makes it easier to build Kotlin Programming and modular UI components. These components can then be mixed to create complex interfaces.
Composable Functions Define UI Elements
Composable functions are the foundation of UI in Jetpack Compose. They outline the UI elements needed. The Kotlin compiler then turns these functions into actual UI components.
Kotlin Compiler Plugin Transforms Composable Functions into UI Elements
The Kotlin compiler plugin is vital in Jetpack Compose. It converts composable functions into UI elements for the Android screen. This lets developers focus on the UI state they want, not the steps to get there.
The Declarative UI Programming of Jetpack Compose makes UI development faster and easier. It helps developers build Android apps that are both responsive and visually appealing.
Previewing Composable Functions
Jetpack Compose is a modern toolkit for Android UI. It has a feature called the @Preview annotation. This lets developers see their composable functions in Android Studio without installing the app. It makes UI design faster and easier.
The @Preview annotation is used on composable functions without parameters. After applying it, previews update instantly with a refresh button click. This saves time and effort by quickly showing UI changes.
Customizing Previews
Android Studio’s Jetpack Compose preview can be customized. Developers can use parameters like:
- widthDp and heightDp to set dimensions
- locale for different languages and regions
- backgroundColor for background colors
- uiMode for UI modes like Night Mode
- LocalInspectionMode for preview customization
Also, the interactive mode lets users test previews. They can check states, gestures, and animations.
The @Preview annotation makes UI development easier with Jetpack Compose and Kotlin.
Composing Rich UI Elements
Jetpack Compose is a modern toolkit for making native Android UIs. It lets developers create stunning and customizable user interfaces. With composable functions and modifiers, they can make UI elements that meet their app’s needs.
Customizing Composable Elements with Modifiers
Modifiers in Jetpack Compose are like a Swiss Army knife for UI customization. They let developers change how elements look, where they are, and how they behave. You can adjust sizes, add padding, and even animations and transformations.
Arranging UI Elements with Composable Layouts
Jetpack Compose has functions like Row, Column, and Box for arranging elements. These layout composables help define how components are organized and placed. This makes for a cohesive and responsive UI.
The declarative nature of Jetpack Compose and its use with Kotlin’s features make UI development easier. It allows for highly customized and dynamic UIs for Android apps. This approach makes creating UIs simpler and more efficient, leading to better user experiences.
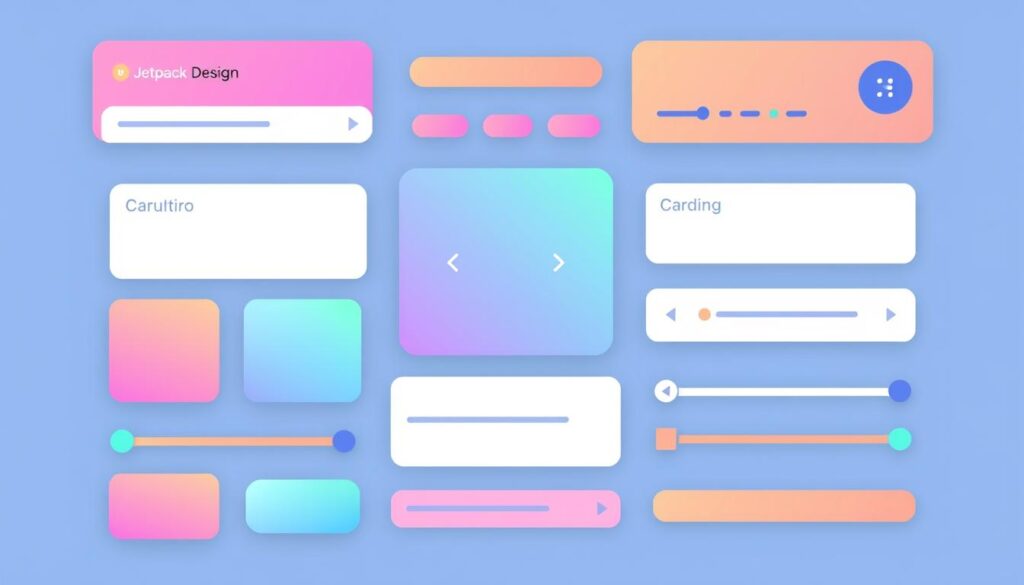
Android App Development Using Kotlin
Kotlin is now a top pick for making Android apps. It lets developers write cleaner, more detailed code. Features like default arguments and lambdas make coding easier. Plus, Kotlin’s builders and DSLs help build complex data structures.
Leveraging Kotlin Features
Kotlin brings many benefits to Android app making. Some key features include:
- Default Arguments: This lets developers set default values for function parameters. It makes code easier to read and less cluttered.
- Lambdas: Lambda expressions in Kotlin make code more direct and clear. They’re great for handling callback functions.
- Data Classes: Kotlin’s data classes automatically create common code. This saves time and effort for developers.
Type-safe Builders and DSLs
Jetpack Compose uses Kotlin’s builders and DSLs to build UIs. This makes creating complex UIs simpler and more intuitive.
Using Android App Development Using Kotlin, Kotlin Programming, Jetpack Compose, and Android Studio helps developers make top-notch Android apps. Kotlin’s features and the Android ecosystem’s tools make app development efficient and easy to maintain. This leads to better user experiences and a smoother development process.
Feature | Benefit |
---|---|
Default Arguments | Reduce the need for overloaded methods, making the code more readable. |
Lambdas | Create more concise and expressive code, particularly when dealing with callback functions. |
Data Classes | Automatically generate common boilerplate code, saving developers time and effort. |
Type-safe Builders and DSLs | Simplify the development process by providing a more intuitive and expressive way to define the app’s UI components. |
Compose Principles and Mental Model
Jetpack Compose is a modern UI toolkit for Android apps using Kotlin. It’s based on declarative UI programming, composition over inheritance, and encapsulation. These ideas help developers make user interfaces that are easy to maintain and grow.
Declarative UI Programming
Jetpack Compose uses a declarative approach. This means developers describe what they want the UI to look like, not how to make it happen. This makes the code easier to read and less prone to mistakes.
Composition over Inheritance
Compose focuses on building UIs from simple parts. This is different from traditional methods that rely on inheritance. It makes it easier to mix and match UI components to create complex layouts.
Encapsulation and Recomposition
Compose’s encapsulation helps keep things organized and makes it simpler to change code. The public API of a composable function is clear, and its inner workings are hidden. The idea of recomposition also plays a big role. It lets Composable functions update the UI efficiently.
By following these principles, Jetpack Compose helps Android developers create better apps. They use declarative UI made from simple, encapsulated functions. This leads to apps that are easier to maintain and grow.
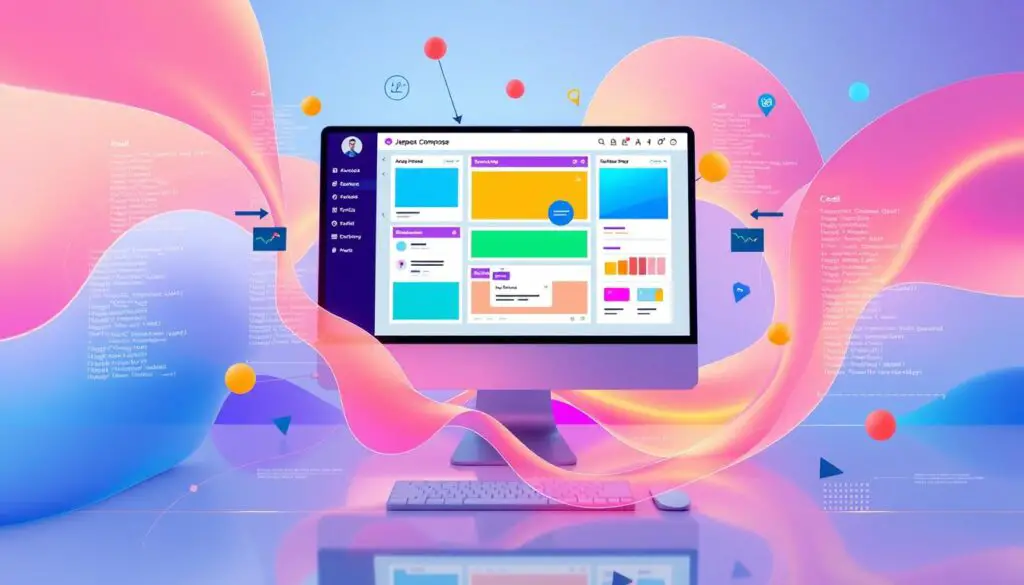
Conclusion
Jetpack Compose and Kotlin have changed how developers make Android apps. Compose’s way of coding and Kotlin’s clear syntax make UI development easier. This leads to apps that are rich, dynamic, and work well.
Jetpack Compose uses new coding ideas to make apps more user-friendly and good-looking. It works well with Kotlin, making coding more efficient. This combo helps developers create better apps faster.
More and more developers are using Jetpack Compose and Kotlin. Those who get good at these tools can make apps that people love. The future of making Android apps looks bright, thanks to Jetpack Compose and Kotlin.