Coroutines are a key feature in Kotlin that makes Android app development easier. They help manage code that runs in the background, keeping the app smooth and responsive. This is because they avoid blocking the main thread.
In Android apps, coroutines are great for tasks that take a long time, like database work or network requests. They turn complex callbacks into simple, sequential code. This makes the app code easier to read and maintain.
The Kotlin Coroutines library offers tools and features for Android app development. Suspend functions are a big part of coroutines. They pause the code until it gets the result it needs, without blocking the thread.
More than 50% of Android developers say coroutines make their work better. They are now a top choice for handling asynchronous tasks in Android. Coroutines offer benefits like light execution, fewer memory leaks, and easy cancellation.
Introduction to Coroutines
What are Coroutines?
Kotlin coroutines are a way to handle tasks that run in the background in Android apps. They were introduced in Kotlin version 1.3. Coroutines are like threads that can pause and start again. This makes them great for handling long tasks without slowing down the app.
Advantages of Using Coroutines in Android Development
Coroutines offer many benefits for Android apps. They prevent tasks from blocking the main thread, keeping the app smooth. Over 50% of professional developers who use coroutines have reported seeing increased productivity. They are also very light, allowing many to run together on one thread.
Coroutines help avoid app freezes by moving tasks off the main thread. This makes the app more responsive. They also make the code cleaner and easier to read by handling tasks in the background.
The kotlinx-coroutines-android library, version 1.3.9, is the best choice for Android projects. Jetpack libraries also support coroutines, making them easy to use in different parts of the app.
Coroutines are perfect for tasks like network requests, database operations, and heavy computations. They make Android development easier by handling tasks in the background.
Coroutines vs. Threads
Kotlin coroutines are much lighter than Java threads. They use less resources, making it easier to handle many tasks at once. This is without using up too much memory or hitting limits.
For instance, starting 50,000 Kotlin coroutines that wait 5 seconds and then print a period uses very little memory. Using threads for the same task would likely cause memory issues or slow down the system.
Threads are managed by the operating system with complex data structures. On the other hand, coroutines are handled by the language runtime with simpler data structures. Threads also take up more memory, several megabytes, while coroutines use only a few kilobytes.
Coroutines can pause and free the thread they’re using. This gives more control over their life cycle and cancellations. This is more efficient than traditional threads, where switching between them can be costly.
Recent tests show that Kotlin coroutines perform well, even on older devices like the Samsung Galaxy S20 FE. While they might have higher latency variance than a thread pool, the difference is usually small for most Android apps. This is unless there are many background tasks running.
Basics of Coroutines
Kotlin coroutines are a key tool for handling tasks that run at the same time in Android apps. They offer a structured way to manage tasks that happen after a delay. The core idea is structured concurrency, which means new coroutines can only start within a certain CoroutineScope.
This scope controls how long a coroutine lasts, preventing them from being lost or leaked. It also makes sure any errors are reported correctly.
Structured Concurrency
Coroutines work under the structured concurrency rule. This rule says an outer scope can’t finish until all its child coroutines are done. It makes handling tasks that run at the same time easier and clearer.
Developers can write asynchronous tasks in a more straightforward way. This makes the code easier to read and understand.
Scope Builders
Developers can also create their own scope using the coroutineScope builder. This builder makes a new scope and ensures it waits for all child coroutines to finish. It lets multiple tasks run together in a suspending function.
Coroutines are light, so they don’t use up too much memory. In fact, JVM threads can be replaced with coroutines, which are less resource-intensive. This means you can start 50,000 coroutines without running out of memory.
Structured concurrency keeps coroutines from getting lost or leaked. It also makes sure any errors are reported and not lost. This, along with coroutines being light, makes them a great tool for Android app development.
They let developers write code for tasks that happen after a delay in a sequential way. This helps handle concurrency and threading issues well.
Android App Development Using Kotlin
Kotlin is now the top choice for Android app development, with over 60% of developers using it. It’s a modern, concise language that makes coding easier and more efficient. This has made it very popular in the Android world.
Kotlin works well with the Android platform and makes handling long tasks easier. This means apps can run smoothly without freezing up. Kotlin coroutines help manage tasks like network calls and database updates. This keeps the app fast and responsive.
Apps made with Kotlin crash less often than those in other languages. This is because Kotlin has strong safety features and better code quality. Over 70 of Google’s apps use Kotlin, showing it’s reliable for Android.
Metric | Value |
---|---|
Kotlin Adoption among Android Developers | Over 60% |
Reduction in Crash Likelihood for Android Apps with Kotlin | 20% |
Reduction in Codebase Size for Google Home team | 33% |
Reduction in Number of NPE Crashes for Google Home team | 30% |
The Kotlin community is growing, and Google supports it well. This includes top-notch tools in Android Studio. These factors have helped Kotlin become a big hit in Android app development.
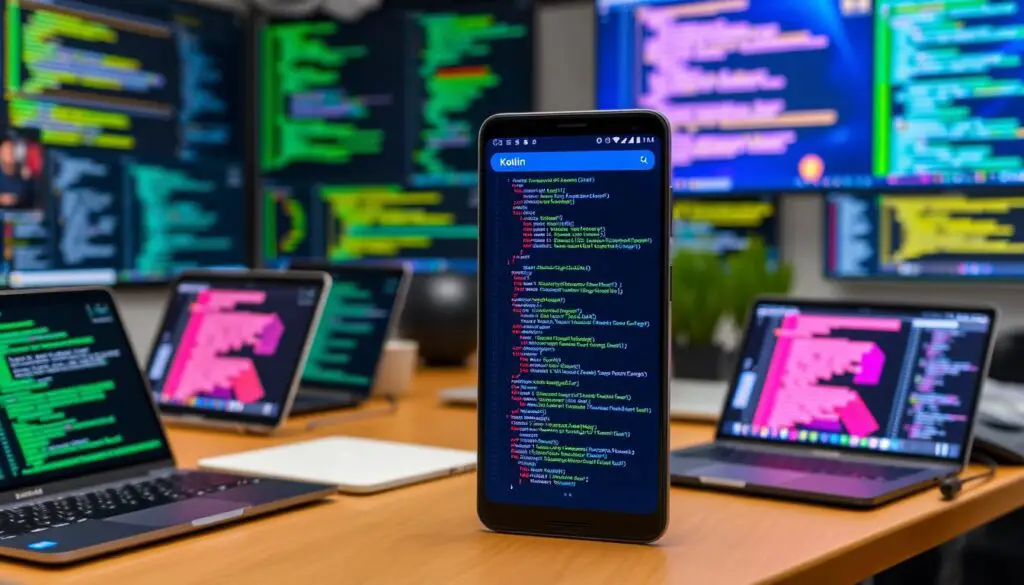
Main-Safety with Coroutines
Kotlin coroutines have changed the game in Android development. They help manage tasks and keep the UI smooth. They ensure that the main thread is not blocked.
Developers use the withContext() function to move tasks to other threads. This keeps the main thread free to update the UI. This makes the app feel more responsive and smooth.
Many blogs talk about the benefits of coroutines. They stress the need to manage Dispatchers to avoid problems. Not handling exceptions can lead to app crashes.
Developers have come up with solutions like safeSuspend and ResultWrapper. These make handling errors easier. The RemoteFailureHandler class helps deal with different exceptions.
By using these strategies, developers can make apps that are safe and efficient. The code examples and instructions help developers improve their skills. They focus on safety and efficiency in Android app development.
Coroutine Scopes and Builders
In Kotlin, coroutines run in a specific CoroutineScope. The launch
builder starts a new coroutine alongside other code. The runBlocking
builder, however, waits until all coroutines in its scope finish. This helps mix regular code with Kotlin coroutines.
launch and runBlocking
The launch
builder is for tasks that don’t need to return anything. It’s easy to start a coroutine and keep going. On the other hand, runBlocking
is for testing or mixing non-suspending code with suspending code.
withContext and suspendCoroutine
The withContext()
function moves a coroutine to a different thread. This keeps the main thread free for UI tasks. The suspendCoroutine
function changes callback-based APIs to coroutines, waiting for the callback to continue.
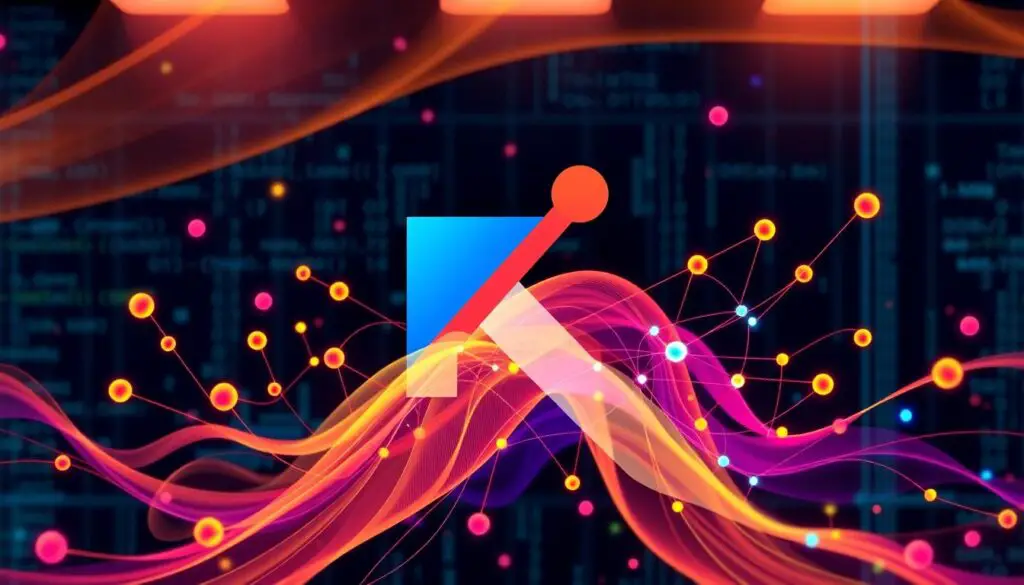
Coroutine Builder | Purpose | Concurrency Handling | Return Value |
---|---|---|---|
launch | Fire-and-forget tasks, tasks without return values | Concurrent execution | Job (no return value) |
async | Tasks that need to return a value | Concurrent execution | Deferred (return a value) |
runBlocking | Bridging non-suspending code with suspending code, testing | Blocks until completion | Result of the block |
Conclusion
Kotlin coroutines have become a key tool in Android development. They make asynchronous programming easier and more efficient. This has greatly improved how apps respond and feel to users.
We’ve seen how Kotlin coroutines help in Android development. They make code simpler, improve how tasks run together, and keep the main thread safe. Developers can use them with Android architecture components like ViewModel. This makes apps better and more responsive.
As Android development keeps changing, Kotlin coroutines will become even more important. Keeping up with the latest in Kotlin coroutines will help developers. They can then create top-notch Android apps that users love and meet industry standards.