How do you use LiveData in Android app development with Kotlin?
LiveData is a special class in Android app development with Kotlin. It knows when to update the app based on its lifecycle. This means it only updates when the app is active.
LiveData uses the observer pattern. This lets activities, fragments, and services get updates and change the UI. It helps make Android apps more responsive and efficient.
LiveData keeps the UI and data in sync. It prevents memory leaks and crashes. It also makes sure data is always up-to-date.
It handles configuration changes well. This means activities or fragments get the latest data right away.
Introduction to LiveData
What is LiveData?
LiveData is a key class in Android app development. It holds data and knows when to update it. It’s like a container for data, like lists, and is often used in ViewModels. It only updates observers who are currently active.
LiveData checks if an observer is active by looking at its lifecycle. If it’s in STARTED or RESUMED, it’s active. This stops inactive observers from getting updates. It helps keep the app stable and prevents memory leaks.
There are special types of LiveData, like MutableLiveData and MediatorLiveData. SingleLiveEvent is for data that only needs to be seen once. These types make working with data easier.
Using LiveData makes updating the UI simpler. It also makes handling changes in screen orientation easier. It’s a big help for Android app developers.
Characteristic | Description |
---|---|
Lifecycle-aware | LiveData automatically handles the lifecycle of its observers, ensuring that data changes are only applied to active app components. |
Observer Pattern | LiveData follows the Observer pattern, allowing observers to subscribe and receive updates when the data changes. |
Lifecycle Owner | LiveData’s observers are associated with a LifecycleOwner, such as an Activity or Fragment, ensuring that they are only notified when the component is in an active state. |
Lifecycle State | LiveData only notifies active observers, i.e., those in the STARTED or RESUMED lifecycle state, about updates, preventing memory leaks and ensuring data consistency. |
Advantages of Using LiveData
LiveData in Android app development with Kotlin brings many benefits. It’s lifecycle-aware, meaning it knows when to start and stop observing data. This prevents memory leaks and keeps the app running smoothly.
It automatically updates the UI when data changes. This makes coding easier and reduces the amount of code needed.
Key Benefits of LiveData
LiveData updates data on the main thread, keeping the UI safe. This lets developers update UI components easily. However, it has limited data transformation options compared to other libraries.
It’s tightly integrated with the Android framework. This makes it perfect for Android projects but harder to use outside of Android.
LiveData has three main types: LiveData, MutableLiveData, and MediatorLiveData. Each type has its own role in updating the UI. LiveData is for observing data, MutableLiveData for setting values, and MediatorLiveData for controlling actions.
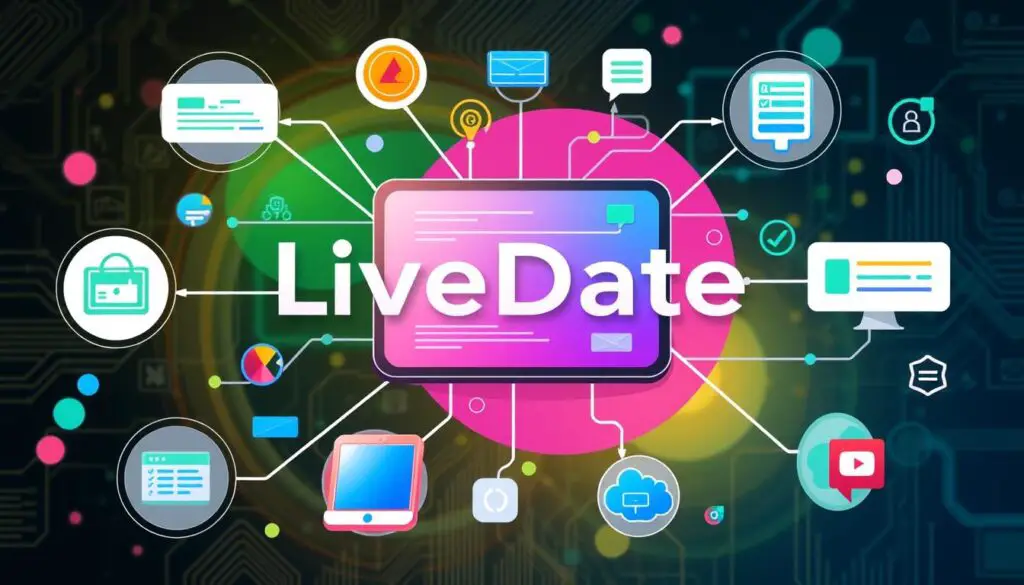
LiveData ensures the UI always shows the latest data. It prevents memory leaks and crashes. It also handles changes like device rotation without manual handling.
Create and Observe LiveData Objects
In Android app development with Kotlin, you need to create and observe LiveData objects. This is key to managing data updates and keeping your UI current. It ensures your app’s state is always up-to-date.
First, you make a LiveData object in a ViewModel class. This object can hold data your app needs to track. For instance, it could be user authentication data or the app’s current state.
-
- Create a LiveData object in your ViewModel class:
private val _authResponse = MutableLiveData<AuthResponse>()
-
- Observe the LiveData object in your Activity or Fragment:
viewModel.authResponse.observe(this as LifecycleOwner) { authResponse -> // Update UI based on the authResponse data }
Next, you create an Observer object. This object has an onChanged()
method. It decides what happens when the LiveData’s data changes. You usually make this in a UI controller, like an Activity or Fragment.
Then, you attach the Observer to the LiveData using observe()
. This method takes a LifecycleOwner object. It makes sure the Observer gets notified of changes, respecting the LifecycleOwner’s lifecycle.
By doing this, you can create and observe LiveData objects well in your Android app. This makes data updates and UI syncing smooth.
Android App Development Using Kotlin
Kotlin is now a top choice for making Android apps. It’s a modern language that’s easy to use and powerful. It works well with Java, letting developers use many Android tools.
Kotlin’s simple code means you need less of it. This makes your code easier to read and work with. It also helps you get things done faster. Features like extension functions and coroutines make coding more efficient.
Kotlin helps avoid bugs by being strict about null values. This makes apps more reliable. It has special operators to handle nulls, preventing common errors.
Google and Android Studio fully support Kotlin. This makes it easy to use Kotlin in your projects. It’s a big plus for developers.
The Kotlin community is always improving the language. It’s great for Android app development because it’s compatible with Java. This makes Kotlin a top pick for modern apps.
Key Advantages of Kotlin for Android App Development
- Concise Syntax: Kotlin’s clean and concise syntax can lead to a significant reduction in the amount of code required, improving productivity and code readability.
- Null Safety: Kotlin’s built-in null safety features, such as the safe call operator (?.) and not-null assertion operator (!!), help developers write more robust and reliable code by preventing common null-related bugs.
- Interoperability with Java: Kotlin seamlessly integrates with the existing Java-based Android ecosystem, allowing developers to leverage the vast array of Android SDK libraries and frameworks.
- Modern Features: Kotlin offers advanced features like extension functions, data classes, and coroutines, which enhance the development experience and enable more efficient asynchronous programming.
- Official Google Support: Google officially supports Kotlin for Android development, with Android Studio providing first-class Kotlin tooling and integration, making it easier for developers to adopt the language.
- Active Community: The Kotlin community is actively contributing to the language’s development, ensuring ongoing improvements and the introduction of new features to benefit Android app development.
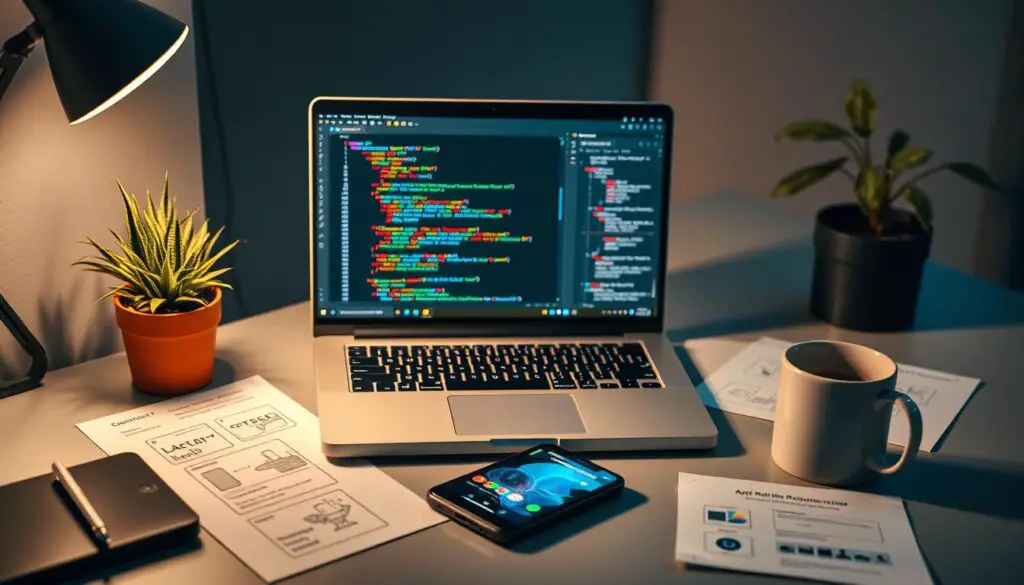
In conclusion, using Kotlin for Android app development has many benefits. It’s easy to use, safe, and works well with Java. Plus, Google supports it, and the Kotlin community is always improving it. This makes Kotlin a great choice for building top-notch Android apps.
Update LiveData Objects
In Android app development with Kotlin, updating LiveData values is key. LiveData doesn’t have direct edit methods. Instead, MutableLiveData offers setValue(T)
and postValue(T)
for changes.
MutableLiveData is often used in ViewModels. ViewModels share only immutable LiveData with observers. To update, use setValue(T)
for the main thread. postValue(T)
is for background threads.
Method | Description |
---|---|
setValue(T) | Updates the data stored in the LiveData object from the main thread. |
postValue(T) | Updates the data stored in the LiveData object from a background thread. |
These methods help update LiveData and alert observers. Always update references to notify observers of new data.
Use LiveData with Room
The Room library supports observable queries. These queries return LiveData objects. They are part of a Database Access Object (DAO) in Room.
Room automatically updates the LiveData object when data changes. This makes it easy to use LiveData with Room. It helps developers observe database changes and update the UI automatically.
Observable Queries with Room
To use LiveData with Room, developers define observable queries in their DAO interface. These queries return LiveData objects. These objects can be observed by UI components like activities or fragments.
When database data changes, the LiveData object updates. This refreshes the UI seamlessly.
Here’s an example of defining an observable query in a Room DAO:
DAO Interface | ViewModel |
---|---|
@Dao public interface UserDao { @Query("SELECT * FROM users") LiveData<List<User>> getAllUsers(); } |
public class UserViewModel extends ViewModel { private final LiveData<List<User>> users; public UserViewModel(UserDao userDao) { users = userDao.getAllUsers(); } public LiveData<List<User>> getUsers() { return users; } } |
In this example, `getAllUsers()` in `UserDao` returns a `LiveData>` object. The `UserViewModel` class exposes this `LiveData` object. UI components can observe it to update the UI when data changes.
Conclusion
LiveData is a key part of the Android Jetpack. It helps developers using Kotlin make apps that are quick and aware of their lifecycle. It uses the observer pattern to keep the UI in sync with the data, avoiding crashes and memory leaks.
Using LiveData in Android app development with Kotlin has many benefits. It handles the app’s lifecycle automatically and keeps the data up to date. It also works well with other Jetpack tools, like Room. This makes building modern Android apps easier and more efficient.
Kotlin is becoming more popular, with over 9.6% of developers using it for Android apps. Its features, like code sharing and a secure environment, make it a great choice. By using LiveData and the Jetpack, Kotlin developers can make apps that are strong, scalable, and user-friendly.