How do you implement local storage in Flutter using SharedPreferences or Hive?
In the world of mobile app development, managing data on the user’s device is key. It makes the app better and faster. Flutter, Google’s open-source UI framework, lets developers use one code for apps on mobile, web, and desktop. This article will look at two popular choices: Hive and SharedPreferences.
Flutter is famous for its Dart programming language and great widgets, UI design, and animation. It’s a top pick for making apps for different platforms. Developers can choose from many data storage options, like SharedPreferences for simple storage or SQFlite for complex data.
Understanding Local Storage and SharedPreferences
Local storage in mobile app development lets apps save data on the user’s device. This is great for apps that need to use important info even without internet. The SharedPreferences plugin in Flutter is a key tool for this.
SharedPreferences is for storing small data like user settings or tokens. It works on many platforms, including Android, iOS, and web apps. You can store int, double, bool, String, and List types of data.
The SharedPreferences plugin has three main APIs: SharedPreferences, SharedPreferencesAsync, and SharedPreferencesWithCache. It’s best to use SharedPreferencesAsync or SharedPreferencesWithCache for better performance and control.
API | Description |
---|---|
SharedPreferences | Provides synchronous access to stored data using a local cache. |
SharedPreferencesAsync | Offers asynchronous access to ensure data consistency and accuracy. |
SharedPreferencesWithCache | Combines the benefits of both synchronous and asynchronous approaches, providing control over caching to suit various storage needs. |
While SharedPreferences is handy for small data, it has its limits. It can face issues with isolates, engine instances, and system preference changes. Developers should pick the right SharedPreferences API for their project’s needs.
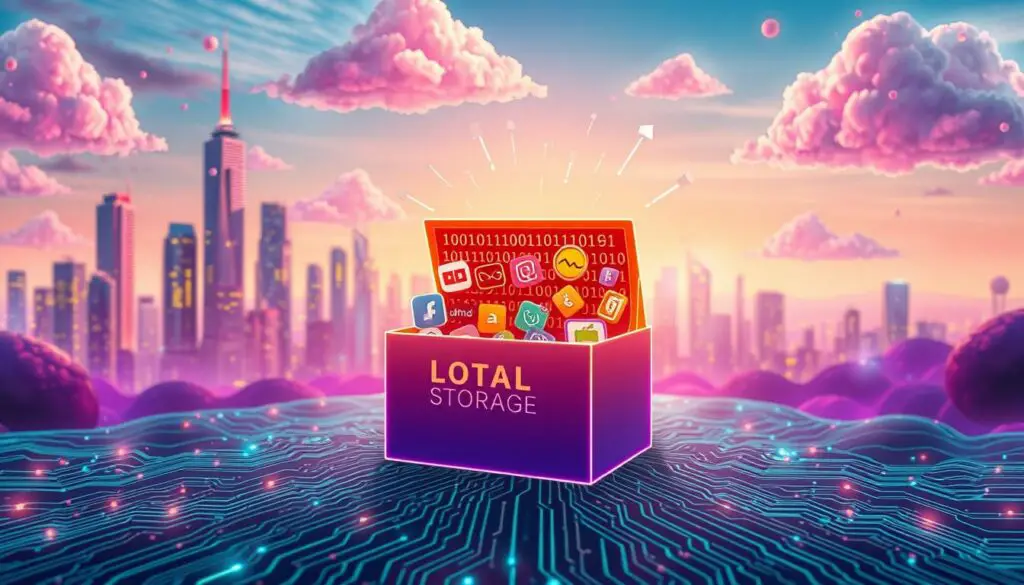
When to Use Local Storage and When to Use SharedPreferences
Flutter developers have several data storage options. For small data like user settings or tokens, SharedPreferences is a top pick.
SharedPreferences is a simple key-value system. It’s great for quick data storage and retrieval. But for bigger or more complex data, Hive might be better.
Hive: A Powerful Local NoSQL Database
Hive is a NoSQL database for Flutter. It’s fast and scalable, perfect for big data. It uses binary serialization for quick data access.
SharedPreferences is easy to use for simple data. But Hive is better for complex data needs. It supports advanced data structures and queries.
Feature | SharedPreferences | Hive |
---|---|---|
Data Types Supported | int, double, bool, String, List<String> | Supports complex data types |
Data Security | Limited security measures | Offers built-in encryption for data security |
Performance | Suitable for small amounts of data | Optimized for high-performance applications |
Querying Capabilities | Basic key-value storage | Supports SQL-like querying and data manipulation |
In summary, SharedPreferences is great for small, simple data. Hive is better for large or complex data. Choose wisely based on your app’s needs.
Implement Local Storage in Flutter using SharedPreferences
The SharedPreferences package is a top pick for storing small data in Flutter apps. It’s great for saving settings, preferences, or tokens. This system is easy to use and keeps data on the user’s device for later.
To start using SharedPreferences in your Flutter app, just follow these steps:
- Add the
shared_preferences
package to yourpubspec.yaml
file. - Initialize the SharedPreferences package in your
main.dart
file. - Use the SharedPreferences API to store and get key-value pairs in your app.
For instance, you can save an integer value of 42
in local storage with SharedPreferences like this:
final prefs = await SharedPreferences.getInstance();
await prefs.setInt('counter', 42);
To get the saved value later, do this:
final prefs = await SharedPreferences.getInstance();
final counter = prefs.getInt('counter') ?? 0;
The code shows how to start local storage, get data, update it, and delete it with SharedPreferences in Flutter. It’s great for keeping data when the app is closed, improving the user experience.
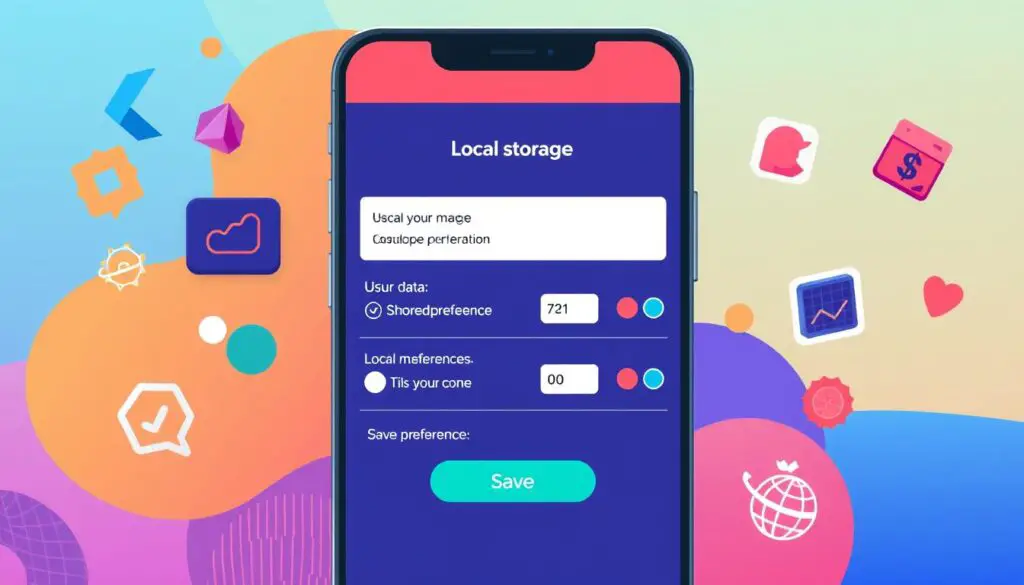
Using the SharedPreferences package lets you create a flexible storage solution for your Flutter app. You can customize it to fit your app’s needs perfectly.
App development using Flutter: Implement Local Storage with Hive
Hive is a top choice for managing large datasets in Flutter apps. It’s a fast, efficient NoSQL database for local storage. It outperforms traditional methods like SharedPreferences in terms of speed and scalability.
Hive’s storage system is fast, making it great for apps with lots of data. It uses binary serialization to make data storage and transmission smaller and faster.
Hive: A Robust NoSQL Database for Flutter
Hive works well offline, allowing apps to run smoothly without internet. It supports many data types, including strings, numbers, and lists. It also handles custom objects with TypeAdapters.
To use Hive in Flutter, add hive
and hive_flutter
to your pubspec.yaml
file. Use flutter pub run build_runner build
to generate type adapters for data handling.
In main.dart
, initialize Hive and create data models. Store them in Hive boxes for easy data management.
Hive makes it easy to add, get, update, and delete data. Use ListView.builder
to display data in UI widgets for a smooth user experience.
Hive supports complex data types and custom adapters. It also has advanced features like queries and transactions. Plus, it offers strong security for sensitive data.
Compared to SQLite and SharedPreferences, Hive is faster, more scalable, and easier to use. It’s a favorite among Flutter developers. Hive’s key-value storage and binary serialization help create efficient apps that work well offline.
Conclusion
Using local storage in Flutter, like SharedPreferences and Hive, boosts app performance and user experience. These tools help store everything from simple preferences to large datasets. They make data storage reliable and efficient.
SharedPreferences is great for simple data needs. It’s lightweight and easy to use. Hive, however, is better for complex data. It offers better performance and grows with your app’s data needs.
Flutter’s popularity is growing fast, with 46% of developers using it. Managing local storage well is key. By learning to use SharedPreferences and Hive, developers can make apps that work smoothly. This makes their apps stand out in a crowded market.