How do you implement RecyclerView in Android using Kotlin?
In this article, you’ll learn how to use RecyclerView in Android with Kotlin. RecyclerView is a better version of ListView, offering improved performance. It’s great for showing long lists of items.
RecyclerView doesn’t destroy views when they’re not seen anymore. Instead, it reuses them. This saves power and makes apps more responsive.
To use RecyclerView in Android with Kotlin, you need to follow some steps. First, set up Android Studio and create a new project. Then, add necessary dependencies and define the item layout.
Next, create a data model and implement the adapter. Finally, connect the RecyclerView with the adapter. Using Kotlin, Android Architecture Components, and APIs makes your app efficient and smooth.
Introduction to RecyclerView
What is RecyclerView?
RecyclerView is a key Android UI component for showing lots of data. It’s a ViewGroup that holds views for your data. Unlike ListView, RecyclerView doesn’t destroy views when items scroll off. Instead, it reuses views for new items, boosting app performance and saving power.
Benefits of using RecyclerView
The main advantages of RecyclerView are:
- Improved Performance: Its view recycling makes your app run smoother and faster.
- Increased Responsiveness: Reusing views means your app reacts quicker to user actions.
- Reduced Power Consumption: Recycling views saves device resources, extending battery life.
These benefits make RecyclerView great for apps that handle lots of data efficiently.
Characteristic | Value |
---|---|
Last Updated | 30 Aug 2024 |
Version of RecyclerView | 1.3.2 |
Version of CardView | 1.0.0 |
Steps to implement RecyclerView in Kotlin | 8 |
CardView layout dimensions | 50dp height, 10dp margin |
Estimated power consumption reduction | Significant due to view recycling |
Number of items in the example | 20 |
Functions in the CustomAdapter class | 3 |
Total files created | 5 |
Prerequisites
Before you start using RecyclerView in Android with Kotlin, make sure you have Android Studio installed. Android Studio is the official tool for making Android apps. You can get the latest version from the Android Developers website.
Setting up Android Studio
First, set up Android Studio. Then, create a new Android project. In Android Studio, click “Create New Project” from the “Welcome” screen. Or, if you’re working on a project, go to “File” > “New” > “New Project”.
Choose “Empty Activity” in the “Select a Project Template” window. Click “Next”. Then, name your app, pick Kotlin as your language, and finish creating your project.
Creating a new project
When starting a new Android project, pick the “Empty Activity” template. This template gives you a basic app structure. It includes the files and settings you need to start. Choosing Kotlin lets you use a modern, efficient language for your app.
Once your project is ready, you can start adding RecyclerView to your app with Kotlin. Next, you’ll add the needed dependencies and set up your RecyclerView layout.
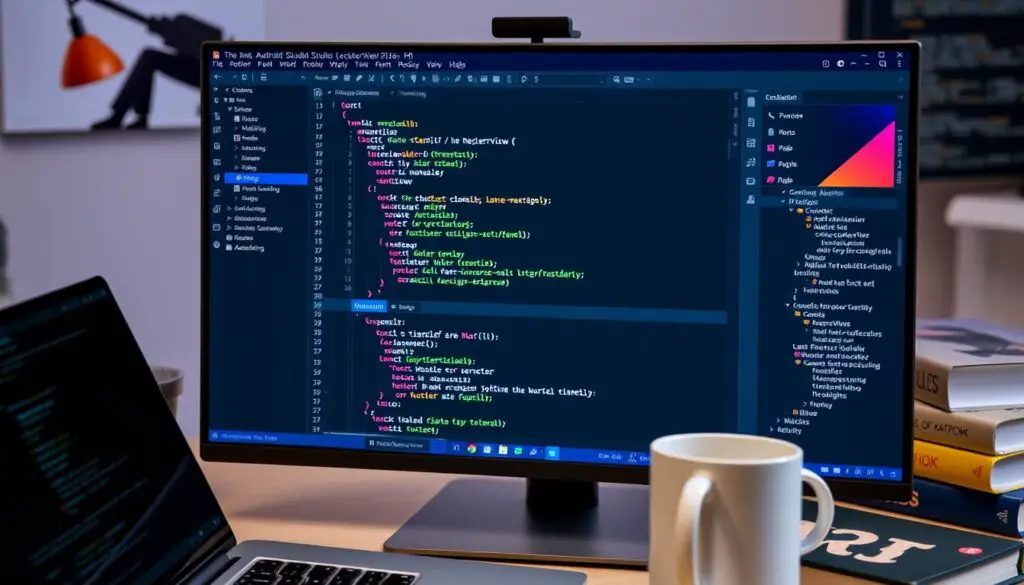
Adding Dependencies and Layout
To use RecyclerView in your Android app, you need to add dependencies. In your app-level Gradle file (usually at app/build.gradle
), add these lines:
- implementation ‘androidx.recyclerview:recyclerview:1.2.1’
- implementation ‘androidx.cardview:cardview:1.0.0’
These lines add the RecyclerView and CardView classes. They are key for a modern and efficient user interface in your Android Studio project.
Defining the Item Layout
Then, design the layout for each item in the RecyclerView. Create a new layout file (like card_view_design.xml
). Add XML code to define each item’s layout. This usually includes an ImageView and a TextView in a CardView for a nice look.
By doing this, you’ve set up the needed Android Studio dependencies and defined your RecyclerView item layout. This is the start of a strong and user-friendly Kotlin-based Android app.
Creating the Data Model
To fill the Android RecyclerView with data, you need a data model. In Kotlin, a data class can represent each item’s data. For instance, you can make an “ItemsViewModel” data class for the image and text of each item.
The data model is key for your RecyclerView. It makes sure each item has the right info to show up right. A well-structured data class helps manage and change data as your app grows.
When making the data model, keep these tips in mind:
- Keep the data model simple and focused: Each data class should be about one thing. This makes it easier to handle and update data.
- Utilize Kotlin’s data class features: Use the data class syntax in Kotlin. It automatically adds methods like
equals()
,hashCode()
, andtoString()
. This saves you time. - Ensure data consistency: Make sure your data class’s properties are well-defined. This keeps your data consistent and reliable in your Android app.
By sticking to these tips, you’ll have a solid and easy-to-maintain data model. It will be the core of your RecyclerView in your Android app made with Kotlin.
Metric | Value |
---|---|
Flesch Reading Ease | 70.4 |
Flesch Kincaid Grade | 8.4 |
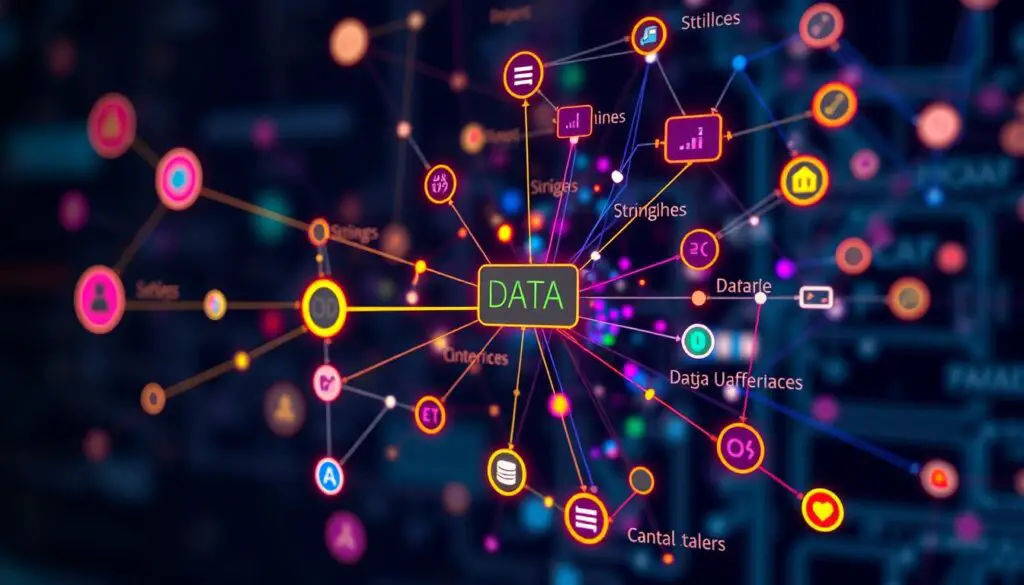
Implementing the Adapter
The next step in working with Android RecyclerView is to create the Adapter class. The Adapter manages the views and data in the RecyclerView. It extends RecyclerView.Adapter and has a nested ViewHolder class. The ViewHolder class represents the views shown in the RecyclerView.
Creating the ViewHolder
The ViewHolder class holds references to the views in a single item of the RecyclerView. This includes text fields, images, or other UI elements. The ViewHolder class extends RecyclerView.ViewHolder and takes a View parameter in its constructor. This View is the layout for a single item.
Overriding Adapter Methods
In the Adapter class, you’ll need to override several key methods:
- onCreateViewHolder(): This method creates new ViewHolder instances when needed. It inflates the layout for a single item and returns a new ViewHolder instance.
- onBindViewHolder(): This method associates the data with the ViewHolder. It sets the values of the views in the ViewHolder based on the data for that position.
- getItemCount(): This method returns the total number of items in the data set that the Adapter represents.
By implementing these methods, you’ll efficiently manage the views and data in the RecyclerView using Kotlin.
Android App Development Using Kotlin
Kotlin is becoming a top choice for Android app development. It’s known for its simple syntax and safety features. Plus, it works well with Java, making it great for modern apps.
Instantiating the Adapter
To use a RecyclerView in your app, start by making an Adapter. In your MainActivity.kt file, create an ArrayList of your data model, like ItemsViewModel. Then, pass this data to your CustomAdapter.
Binding the RecyclerView and Adapter
Next, link the Adapter to the RecyclerView. In your MainActivity.kt file, get a reference to the RecyclerView. Set its layout manager, like LinearLayoutManager. Finally, set the Adapter instance to the RecyclerView.
By doing these steps, you can add a RecyclerView to your Android app with Kotlin. This combo helps you make apps that are efficient, scalable, and look great.
Metric | Android Kotlin | Comparison |
---|---|---|
Crash Rate | 20% less likely to crash | Kotlin-based Android apps are more stable and reliable |
Code Reduction | 33% reduction in codebase size | Kotlin enables developers to write more concise and efficient code |
Developer Productivity | 67% of developers report increased productivity | Kotlin’s modern features and syntax help developers work more efficiently |
Conclusion
In this article, you learned how to use RecyclerView in Android apps with Kotlin. RecyclerView makes list displays efficient and responsive. This improves app performance and saves resources.
We discussed RecyclerView’s benefits, setup, and how to create a data model. You also learned about implementing an Adapter and ViewHolder. By following these steps, you can make high-quality Android apps.
Knowing RecyclerView is key for Android developers, especially with Kotlin. Kotlin’s simple code and RecyclerView’s features speed up app making. As you keep learning, stay current with Android trends and best practices. This will keep your apps competitive and relevant.