How do you manage navigation between activities in Kotlin?
Creating Android apps with Kotlin means making sure users can move easily between screens. This is key for a smooth user experience. Developers use the Navigation component from Android Jetpack to make this easier in their Kotlin projects.
The Navigation component makes it simpler to set up how screens move from one to another. It lets developers manage these transitions well. This way, they can make their apps more user-friendly and quick to use.
In this article, we’ll dive into how to navigate between activities in Kotlin Android apps. You’ll see how to use the Navigation component, set up navigation graphs, and handle dynamic arguments. This will help you create top-notch mobile experiences with Kotlin.
Introduction to Navigation in Android Apps
The Importance of Effective Navigation
Navigation is key for a smooth user experience in Android apps. Users want easy transitions and quick access to content. Bad navigation can make users unhappy and leave your app.
Overview of the Navigation Component
The Navigation Component is part of Android Jetpack. It makes managing complex navigation easier. It helps with animations, deep linking, and passing data between screens.
This component uses a Fragment-Based Architecture. It keeps UI logic for each screen in Fragments. This makes your app’s navigation better to manage and more flexible.
- Handling Fragment transactions and standardized animations
- Facilitating the implementation of deep linking and Navigation UI patterns like Navigation Drawer or Bottom Navigation Views
- Providing easy integration with ViewModel and other Android Jetpack components
Using the Navigation Component makes your Android app’s navigation better. It leads to happier users and more engagement.
Migrating to a Fragment-Based Architecture
To get the most out of the Navigation component, moving your Android app to a fragment-based architecture is a good idea. This means taking the UI logic for each screen out of activities and putting it into fragments. This way, each activity only deals with the global navigation UI, making your code easier to manage and maintain.
Moving Screen-Specific UI Logic to Fragments
For a successful switch to a fragment-based architecture, separate the UI logic for each screen into its own fragment. This keeps your activities focused on the big picture of navigation. Meanwhile, fragments handle the details of each screen’s UI and interactions. This makes your code more organized and simpler to handle.
Creating a Fragment Host Layout
After moving the UI logic to fragments, the next step is to set up a fragment host layout in your activities. This layout holds all the fragments that make up your app’s navigation. It makes switching between screens smooth and easy, without the hassle of complex navigation logic.
Benefit | Description |
---|---|
Fragment-Based Architecture | Separates screen-specific UI logic from the overall navigation structure, improving maintainability and flexibility. |
Activity Refactoring | Allows activities to focus on managing the global navigation, while fragments handle the specific UI elements and interactions. |
UI Logic Separation | Promotes a more modular codebase by isolating the UI logic for each screen into its own fragment. |
Android Fragments | Provides a flexible and reusable way to manage the UI components of your Android app. |
Activity Host Layout | Serves as a container for the various fragments, enabling seamless navigation between screens. |
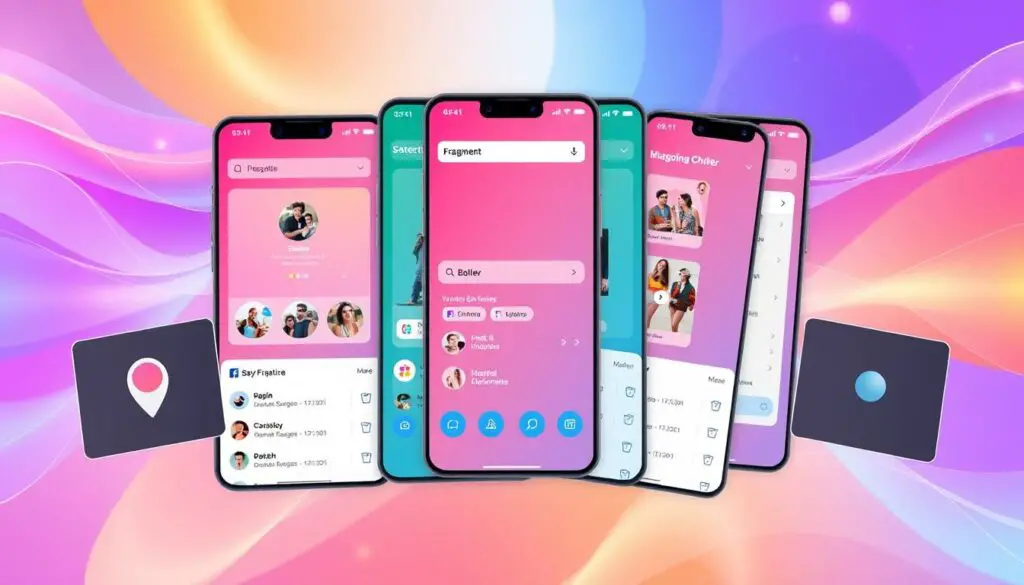
Integrating the Navigation Component
Navigating between screens in an Android app is key to a good user experience. The Android Jetpack Navigation component makes this easier. It offers a standard, efficient way to manage navigation in your app. This makes your app’s navigation smooth and easy for users.
The Navigation Graph is a big part of the Navigation Component. It shows all the destinations (screens or fragments) in your app. It also shows how to get from one to another. Setting up the Navigation Graph Setup is important for using the Navigation Component in your Android app.
- First, create a new
navigation
resource file in your Android project. This file is the heart of your navigation graph. - In the Navigation Editor, you can add your app’s destinations. These can be fragments, activities, or even other navigation graphs.
- Then, define how users can move between these destinations. This is done by setting up actions for navigation.
- Finally, add the
NavHostFragment
to your app’s layout. It will show the right destination based on user actions.
With the Navigation Graph Setup done, you can use the Android Jetpack Navigation component. It makes Navigating Between Screens in your app easy. The Navigation Controller helps manage the flow of navigation. It makes it simple to move between destinations and handle the back button.
Using the Navigation Component Integration makes your app’s navigation better. It creates a smooth, user-friendly experience. This tool from the Android Jetpack suite makes navigation easy. It lets you focus on making your app engaging and seamless for users.
Android App Development Using Kotlin
In the world of Kotlin Android Development, the Navigation component is a key tool. It helps manage how users move through your app. With Navigation Graphs, developers can map out how the app should flow, making it easy for users to navigate.
Setting Up Navigation Graphs
The heart of the Navigation component is the Navigation Graphs. These graphs show how different parts of your app connect. Using Jetpack Navigation, you can set up these paths and transitions easily. This makes it simpler to navigate your app with Kotlin.
To start, you need to create a navigation file in “res/navigation”. This file will outline your app’s navigation, including all the fragments and activities.
Feature | Benefit |
---|---|
Navigation Graphs | Provides a visual representation of your app’s navigation structure, simplifying the development process. |
Fragment Navigation | Allows you to manage the navigation between different fragments within your app, enhancing user experience. |
Jetpack Navigation | The official navigation component provided by Google, enabling seamless Kotlin Android Development. |
Navigating with Kotlin | Leverages the power of the Kotlin programming language to streamline the navigation implementation in your Android app. |
Navigating Between Fragments
With your Navigation Graphs ready, you can now focus on moving between fragments. The Navigation component offers tools and APIs to help. You can define actions, handle arguments, and smoothly move between fragments.
Using Jetpack Navigation libraries, you can write clean and efficient Kotlin code. This ensures a smooth and consistent user experience in your app.
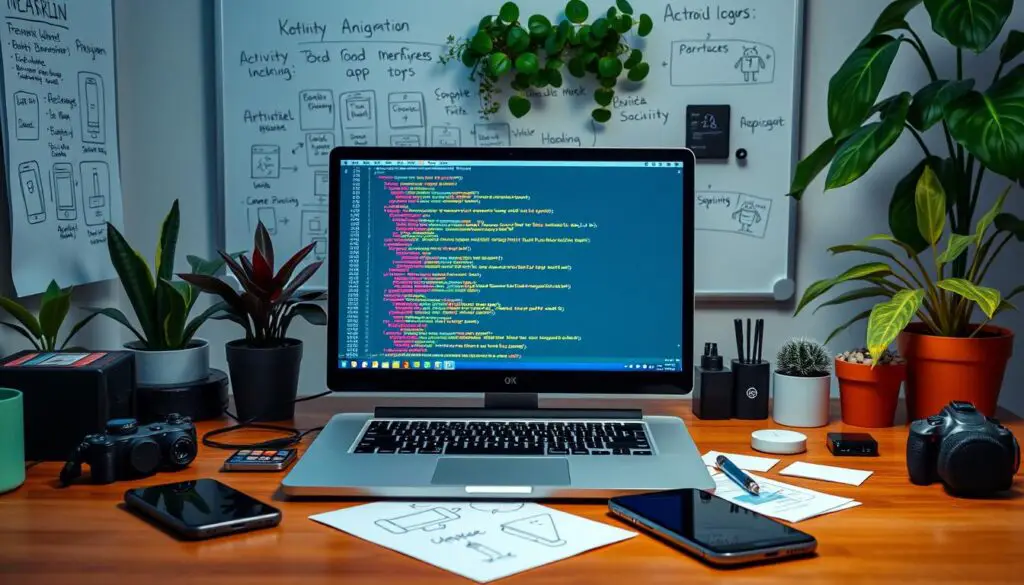
Adding Activity Destinations
The Navigation component is great for navigating between fragments. But it also works for activity destinations. This guide will show you how to add activity destinations to your navigation graph. You’ll learn how to configure them and handle dynamic arguments.
Configuring Activity Destinations
Creating an activity destination is similar to making a fragment destination. You use the same createGraph()
lambda. The Navigation library links the NavController
to an Activity layout. This layout is where the navigation graph is tied to the active Activity.
An Activity destination is like an endpoint in your navigation graph. Switching to a different Activity means the current navigation graph is no longer valid. You’ll need to manage the navigation flow carefully.
Handling Dynamic Arguments
The Navigation component lets you use dynamic arguments in both activity and fragment destinations. You can use the dataPattern
attribute to add named placeholders in the URL. This lets you swap in dynamic data, like user IDs, when you navigate.
In some cases, you might not want to depend on the activity class at compile-time. Using implicit intents could be a better choice. The intent-filter
in the destination Activity‘s manifest helps set up the Activity destination.
Remember, including arguments in your navigation requests is key. If you forget a mandatory argument, an exception is thrown. Also, if you try to navigate to a destination and the app is missing, or the destination app doesn’t have an Activity that matches the intent-filter
, an ActivityNotFoundException
is raised.
Conclusion
In this article, we looked at how to manage navigation in Android apps made with Kotlin. Kotlin is a modern language that’s popular in Android development. We talked about using the Navigation component, a tool from Android, for smooth transitions between screens and activities.
Using the Navigation component helps developers manage complex navigation flows easily. It makes sure the app is easy to use. We also talked about using a fragment-based architecture. This makes apps more modular and scalable.
As you keep making Android apps with Kotlin, it’s important to know the latest Android Navigation Best Practices and Kotlin Android Development Techniques. Using the Navigation Component Benefits helps create Seamless User Experiences. This article has given you the tools to excel in Kotlin-powered Android app development.