What are widgets in Flutter and how are they used?
Flutter is Google’s open-source framework for making mobile apps. It has changed how developers create apps for different platforms. At the heart of Flutter apps are widgets, which are the basic building blocks of the user interface.
Widgets in Flutter handle the visual parts of the app, user interactions, and the app’s state. Flutter has 14 types of widgets, each with its own role in an app. These range from simple text and images to complex layouts and interactions.
Stateless Widgets don’t change once they’re created. They’re good for static content or UI parts that don’t need updates. On the other hand, Stateful Widgets can change and are used for user interactions, data changes, and evolving app visuals.
A Flutter app’s structure is a tree of widgets, with each widget having child widgets. This tree makes it easy to build and reuse UI parts. Developers use Dart to create powerful, scalable apps with Flutter.
Introduction to Flutter Widgets
Flutter is an open-source framework for making mobile apps. It uses widgets as the core of its design. These widgets are the building blocks of a Flutter app’s UI.
Widgets describe a part of the UI and can be quickly added to the render tree. This makes Flutter’s UI rebuild smoothly when changes happen. It’s all about Flutter performance optimization.
Flutter’s Reactive Architecture
Flutter’s UI is rebuilt when the widget tree changes. This makes building UIs easier and more declarative. Developers just need to describe the UI based on the app’s state.
Flutter’s reactive nature keeps the UI in sync with the app’s data. This reduces the need for manual UI updates. It makes app development simpler.
Immutable Nature of Widgets
Flutter widgets are immutable. They have no mutable state, as all fields are marked as final. This makes Flutter testing and debugging easier.
If you need mutable state, use a StatefulWidget
. It creates a State
object for dynamic behavior.
Widgets’ immutability and Flutter’s reactive design make for an efficient app architecture. Developers can focus on building reusable widgets. This leads to a maintainable and scalable codebase.
The Widget Tree
At the heart of Dart programming and Flutter UI design are widgets. These are the basic parts for making mobile apps. In mobile app development with Flutter, everything – from buttons to full apps – is a widget. They form a tree-like structure called the “widget tree,” showing who’s in charge of whom.
The widget tree changes when something new happens. This lets Flutter update the UI quickly and smoothly. It’s key to making the app look good and work fast. Knowing how to use the widget tree helps with managing the app’s state and handling user actions.
Widget Composition and Nesting
Flutter is great at making complex UIs by mixing different widgets. By putting widgets inside other widgets, developers can make beautiful designs. This is how you build strong and growing Flutter apps.
To make apps run better, use const and variables wisely. As apps get bigger, using tools like Provider or Bloc helps keep things stable and fast.
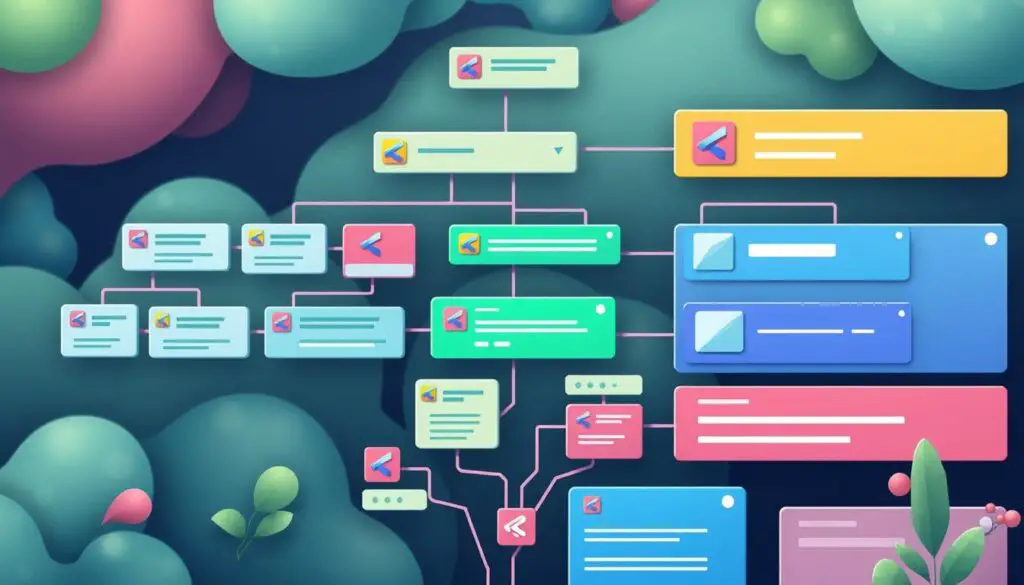
Getting the widget tree is key for Dart programming, Flutter UI design, and mobile app development with Flutter. Learning how to mix and nest widgets, and manage state well, lets developers make apps that are fun to use.
Stateless Widgets
In the world of app development using Flutter, stateless widgets are key. They don’t change and are used for things like text labels and buttons. These widgets are important for creating cross-platform apps.
Stateless widgets have a build
method that makes a new widget tree. When something changes, this method is called again. This makes the app run smoothly and efficiently.
Optimization Technique | Description |
---|---|
Minimizing nodes | Reducing the number of nodes created in the widget tree can improve performance. |
Using const widgets | Leveraging constant widgets and constructors can enhance efficiency. |
Refactoring to stateful | Considering refactoring a stateless widget into a stateful widget can be beneficial in certain scenarios. |
Stateless widgets in cross-platform app development with Flutter are part of the Widget
class. They are simple to use and don’t change. The build
method makes the widget based on its properties.
Examples of stateless widgets include ButtonBar
, ListTile
, and IconButton
. These widgets help developers build app development using Flutter with lots of functionality and design options.
Stateful Widgets
In Flutter, stateful widgets are key for dynamic and interactive user interfaces. They can change their internal state over time. This is vital for UI elements that need to update based on user actions or data changes.
Widget Lifecycle Methods
Stateful widgets go through a lifecycle with several methods. These include initState, build, and dispose. These methods help developers set up data, render the widget, and clean up resources when needed.
The initState method is called when the widget is first created. It’s perfect for one-time tasks like setting up a database connection. The build method creates the widget’s visual look. The dispose method is called when the widget is removed, allowing for resource cleanup.
Understanding how to manage a widget’s state is essential for creating high-performance Flutter applications. By knowing the lifecycle and using the right methods, developers can build scalable Flutter app architectures. These can integrate third-party APIs and improve performance.
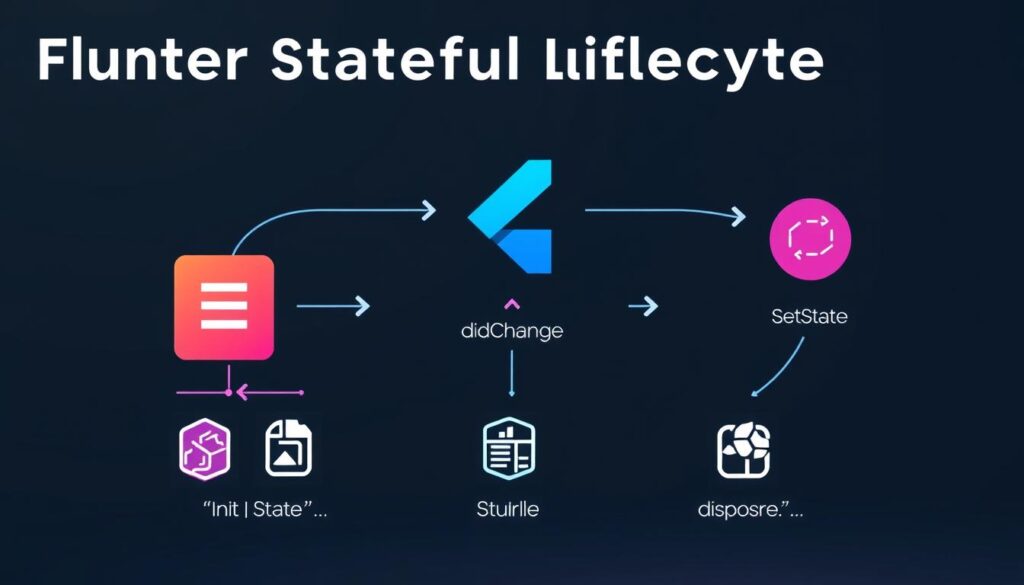
The YellowBird and Bird examples show how to manage and update internal state. They highlight the power and flexibility of stateful widgets in Flutter development.
App development using Flutter
Flutter is a mobile app development framework made by Google. It changes how developers make apps for different platforms. With Flutter, you can create apps for iOS, Android, and the web from one codebase. This makes Flutter popular among developers worldwide.
Flutter’s main strength is its reactive architecture and immutable widgets. These widgets have complex features like scrolling lists and sliders. This lets developers focus on the app’s logic, not the basic parts.
Flutter’s hot reload feature is a big advantage. It lets developers see changes right away without restarting the app. This fast development, along with Dart programming and a reactive model, makes Flutter great for creating engaging apps.
Flutter also has a graphics engine called Skia. It makes animations smooth, even on lower-end devices. Flutter has a big community, detailed docs, and lots of packages. This makes it a full ecosystem for app development using Flutter, cross-platform app development, and mobile app development with Flutter.
Flutter is better than other frameworks like React Native. It has a unified look, better performance, and a faster development cycle. Flutter Flow is a tool for visual app design, making it easier to work together.
The app market is growing fast, and there’s a big need for fast, unique interfaces. With its features and growing community, Flutter is a top choice for businesses. They want to develop apps using Flutter and give users great experiences on many platforms.
Widget Properties and Customization
In Flutter, widgets are key for making user interfaces. They have many customizable properties. This lets developers tweak their look and feel to fit their design needs.
By using these properties, developers can make interfaces that look great and work well. They follow the design rules of the platform they’re on.
Material Design and Cupertino Widgets
Flutter has two main design systems: Material Design and Cupertino. Material Design widgets look sleek and modern, perfect for Android. Cupertino widgets, on the other hand, offer a clean look for iOS.
These widgets help developers make interfaces that feel right at home on the device. They use pre-made UI components to build complex layouts easily.
Material Design Widgets | Cupertino Widgets |
---|---|
AppBar, BottomNavigationBar, Drawer | CupertinoNavigationBar, CupertinoTabBar, CupertinoSliverNavigationBar |
Buttons, Chip, Checkbox, Radio, Switch | CupertinoButton, CupertinoSwitch, CupertinoSegmentedControl |
TextField, DropdownButton, Slider | CupertinoTextField, CupertinoDatePicker, CupertinoSlider |
Using these widgets, developers can make Flutter widgets that fit perfectly with the Flutter UI design. They offer a native user experience, no matter the platform.
Building Complex UIs with Widgets
Flutter is a framework for making apps that lets developers create stunning UIs. It uses a wide range of widgets to build complex designs. These designs fit well on different screens and orientations.
Layout Widgets and Structuring
Flutter’s UI design is centered around layout widgets like Column, Row, Stack, and Expanded. Developers use these to make detailed and attractive UIs for their apps. The way these widgets are arranged is key to making UIs that work well on all screens.
Flutter makes UI development easier by letting developers focus on what they want to achieve. The framework then takes care of making it happen. This way, developers can reuse code and design in a modular way. This is great for big apps.
Knowing how Flutter’s app architecture works is important. It helps manage state, improve performance, and keep code organized. Flutter’s layout system lets developers create custom UIs. Learning advanced layout techniques can make apps run smoother and faster.
Widget Type | Description |
---|---|
Stateless Widgets | Immutable widgets that describe a part of the UI which remains constant throughout the lifecycle of an app. |
Stateful Widgets | Dynamic widgets that can change during runtime and hold mutable state, suitable for UI elements that need to change based on user interactions. |
Using Flutter’s widgets and layout features, developers can make UIs that are both beautiful and responsive. These UIs engage users and offer a great app experience across different platforms.
Conclusion
Flutter has become a top choice for app development. It offers a smooth, native-like experience on many platforms. Developers can make beautiful, fast mobile apps with just one codebase. This makes app making easier and cheaper.
Flutter is used by nearly half of all developers worldwide. This shows how versatile and beneficial it is. Its strong community, detailed guides, and ongoing updates help developers use Flutter to its fullest. They can build apps for Android, iOS, desktop, and web.
The Flutter world is growing, with new features and tools coming out. This makes Flutter a top pick for making mobile apps. It focuses on design, speed, and working on different platforms. This helps developers make amazing user experiences and push app development forward.