How does the useState hook work in React Native?
React is a top choice for making web and mobile apps. The introduction of Hooks in React 16.8 changed how developers handle state in function components. The `useState` hook is a key part of this, helping you add state to your React Native apps.
Knowing how the `useState` hook works is key for making apps with React Native. This article will cover the `useState` hook in detail. We’ll look at its uses and advanced topics to help you manage state in your React Native projects.
Understanding the Need for State Management
Effective state management is key in cross-platform app development and mobile app frameworks like React Native. Without it, your app’s data flow can get messy. This leads to bugs, slow performance, and development headaches. The `useState` hook helps manage state in your functional components. It keeps your app fast and user-friendly.
The Importance of Proper Implementation
React focuses on a component’s internal state for managing state. Before Hooks, class components were used. Now, with `useState`, function components can handle state too. This makes your code easier to read and maintain.
React’s Approach to State Management
The `useState` hook is a big step forward in React Native components, React Native navigation, and React Native styling. It makes state management easier and faster. This ensures your app works well, even when it’s busy.
React Hooks, like `useEffect`, are important for handling side effects and improving React Native performance optimization. Custom hooks help by making stateful logic reusable. This keeps your code organized and easy to manage.
Introducing the useState Hook
What is a Hook?
Hooks are a new feature in React, introduced in version 16.8. They let you use React features like state and lifecycle methods without a class component. The `useState` hook is very popular because it lets you add state to functional components.
The useState Hook Explained
The `useState` hook is a function that takes an initial state value. It returns an array with the current state and a function to update it. This makes managing state in functional components easy, just like in class components.
With `useState`, function components can have state, making them more like class components. You can use `useState` to add state to your components. It keeps the state between updates, handling different types like strings, numbers, and objects.
React doesn’t update the state right away after calling `setMessage(). This shows how it works asynchronously. Also, React batches multiple `setState` calls, updating only once in React event handlers. But, if `setState` is called outside React, it will update each time.
Understanding and using `useState` well can make managing state easier. Trying out examples can help you grasp its behavior better.
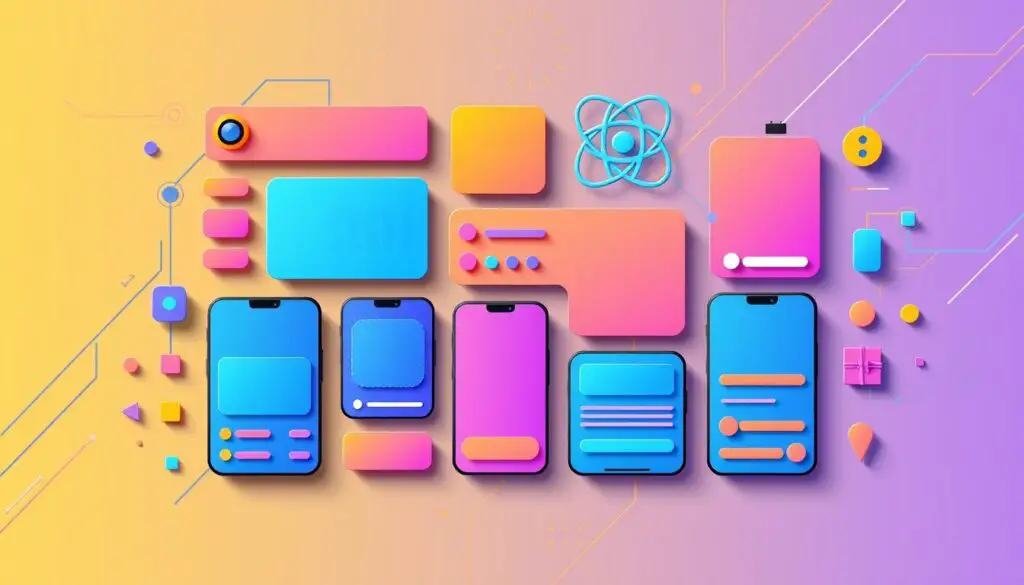
Key Fact | Value |
---|---|
React Native Support for Hooks | Since the 0.59 release |
Hooks Introduced in React | 16.8.0 |
Hooks Adoption | Opt-in and backward-compatible |
Develop applications using React Native
React Native is a powerful tool from Facebook. It lets developers make high-quality mobile apps with JavaScript and React. The `useState` hook helps manage state in components, making apps smooth and consistent on all platforms.
The `useState` hook is key for making strong mobile apps. React Native lets developers build apps for Android and iOS with one codebase. This saves time and money.
To start with React Native, using Expo is recommended. Expo offers tools and utilities to make development easier. It also makes upgrading React Native versions simpler, which is why it’s the top choice for developers.
Developers need to know JavaScript well to work with React Native. For Android apps, having the right Java SDK version is crucial. React Native’s Gradle tool needs a specific setup.
To create a new React Native project, `npx` is used. It sets up a project with a ready `App.js` file. Visual Studio Code and Android Studio are the best code editors for React Native projects.
React Native Feature | Description |
---|---|
React Native Components | Reusable building blocks that make up the user interface of a React Native application. |
React Native Navigation | Enables seamless navigation between different screens and views within a React Native app. |
React Native Styling | Allows developers to style their React Native components using CSS-like syntax. |
React Native Performance Optimization | Techniques and tools to ensure smooth and responsive performance in React Native applications. |
React Native Debugging Tools | Utilities and frameworks that aid in the debugging and troubleshooting of React Native apps. |
React Native Testing | Frameworks and methodologies for writing and executing tests to ensure the quality of React Native applications. |
React Native Deployment | Processes and tools for packaging, distributing, and deploying React Native applications to app stores and other platforms. |
By learning the `useState` hook and React Native’s features, developers can make many types of mobile apps. These apps offer a great user experience, no matter the platform.
Working with useState
The useState
hook is a key tool for managing state in React Native components. It lets you declare and initialize state variables easily. You can also read and update them as needed.
Declaring and Initializing State Variables
To use useState
, first import it from ‘react’. Then, call useState
with an initial value for your state variable. It returns an array with the current state and a function to update it.
For example, managing a counter in your app:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
// ...
}
In this example, count
is the current counter value. The setCount
function updates it.
Reading and Updating State
After declaring a state variable with useState
, you can read and update it. To read, use the state variable in your JSX. To update, call the update function with the new value. React will then update your component.
Here’s how to update the counter state:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<View>
<Text>The count is: {count}</Text>
<Button
title="Increment"
onPress={() => setCount(count + 1)}
/>
</View>
);
}
In this example, the count
state is displayed. Pressing the “Increment” button updates it, causing the component to re-render.
Mastering useState
helps manage your React Native components’ state. This ensures a smooth user experience.
Advanced useState Concepts
Mastering the `useState` hook in React Native is more than just basic use. Developers must explore its deeper concepts to fully utilize it. Understanding the asynchronous nature and batching of state updates are key.
Asynchronous Nature of useState
When you update the state with `useState`, React doesn’t change it right away. It adds the update to a queue and updates the component later. This is important to remember, especially if you need the new state value right away.
Batching Multiple State Updates
React batches multiple state updates together. This means it only updates the component once, not after each update. This makes your app faster and more efficient. But, you can stop batching in some cases, like with `setState` outside React events.
Knowing these advanced concepts helps developers make better apps. They can make their apps faster and more user-friendly. By using `useState` and batching updates wisely, developers can create top-notch React Native apps.
Integrating useState with Other Hooks
The useState hook works well with other React Hooks like useEffect, useContext, and useReducer. Using these Hooks together boosts your React Native app’s state management. This makes your app more complex and user-friendly. It lets you handle side effects, share state, and use advanced state management.
Combining useState and useEffect
Using useState and useEffect together is great for fetching data from APIs. The useState hook manages the data state. The useEffect hook fetches the data when needed. This keeps your React Native components updated without manual updates.
Integrating useState and useContext
The useContext hook helps access context data in functional components. Combining it with useState creates a centralized state system. This is useful for managing global state, like user settings or themes.
Leveraging useState with useReducer
The useReducer hook is great for complex state management with useState. It uses a reducer function for detailed state changes. This makes your React Native app’s state management more structured and scalable.
Learning to use useState with other React Hooks helps build better React Native apps. These apps manage state well and offer a smooth user experience.
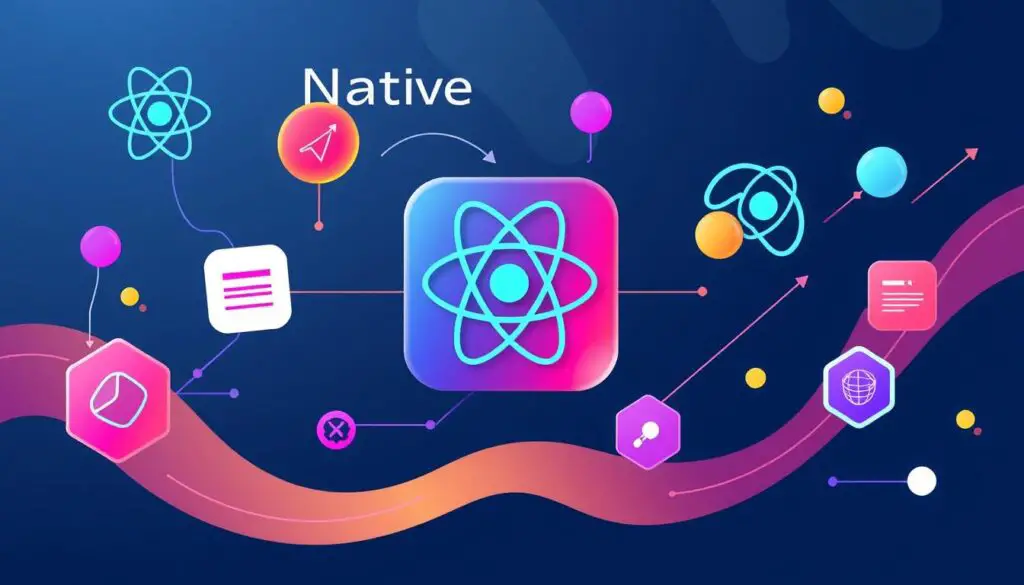
Conclusion
In this article, we’ve looked at the basics of the `useState` hook. It’s key for managing state in React Native apps. By learning how to use `useState`, you can make your React Native apps more responsive and efficient. You’ll also find out how to improve your app’s performance and debug it better.
The `useState` hook is a powerful tool for app developers. It helps manage state well, leading to better user experiences. React Native is popular because it works well on different platforms and is easy to learn.
Mastering the `useState` hook and the React Native framework lets you create amazing mobile apps. You can use React Native’s components, navigation, and styling to make your apps stand out. This way, you can build apps that are both innovative and user-friendly.