How do you create a functional component in React Native?
React Native is a popular framework for making mobile apps with JavaScript and React. It uses components, like View and Text, to build the UI. These components can be nested to create complex interfaces.
React Native’s components are based on React, a JavaScript library for UIs. React uses a declarative approach, where you describe the UI, and React updates it. This makes mobile apps feel and perform like native ones.
React Native is great for creating functional components. These are simple JavaScript functions that return JSX. They are easy to understand, test, and maintain because they focus on rendering the UI.
To make a functional component, just define a JavaScript function that returns JSX. For example, you can create a Button component. It can have customizable text, color, and size. You can change how it looks and behaves by passing props.
React introduced Hooks in version 16.8, making functional components even better. Hooks like useState let you manage state in functional components. This makes them more powerful and flexible.
As developers use more functional components and Hooks, React Native is becoming more popular. Its simplicity, maintainability, and performance are making it a top choice for mobile app development.
Introduction to React Components
At the heart of any React Native app are its components. These blocks are the basic parts of the user interface. Whether you’re developing applications using React Native, exploring mobile app development, or diving into cross-platform development, knowing about components is key.
Understanding Components
In React Native, everything you see is a component. These can be Functional Components or Class Components. Functional Components are simple JavaScript functions. They are often called “Stateless” because they can’t manage their own state or lifecycle methods.
Functional components mainly focus on showing the user interface, not complex actions.
Class Components, on the other hand, are JavaScript ES6 classes that extend React.Component
. They are called “Stateful” because they can handle their own state and lifecycle methods. Class components offer more control over the app’s state and actions.
Components in React Native are vital for mobile app development and cross-platform development. They make it easier to build, keep up, and grow your React Native applications. Knowing the difference between Functional and Class Components helps you choose the right one for your needs.
Functional Components in React Native
React Native is a top choice for making mobile apps. Functional components are key in React Native. They make it easy to build apps for both iOS and Android.
These components are the building blocks of your app. They help shape its structure and how it works.
Defining Functional Components
Functional components in React Native are simple JavaScript functions. They can be written as arrow functions or the traditional function keyword. Unlike class-based components, they don’t manage their own state or lifecycle methods.
They focus on showing the UI based on the props they get. Props are like input values that change how the component looks and acts.
To make a functional component, start with a JavaScript function. This function should return a JSX element. This element is what the component will show on the screen.
The function can take in props. These props let you customize the component’s look and behavior.
Rendering Functional Components
After making a functional component, you can use it in your app. Just call its name as a custom HTML tag in your JSX code. This makes it easy to add the component to your app.
Functional components are very flexible. They can be used for everything from simple buttons to complex screens. By using them well, developers can make apps that are powerful, responsive, and easy to keep up.
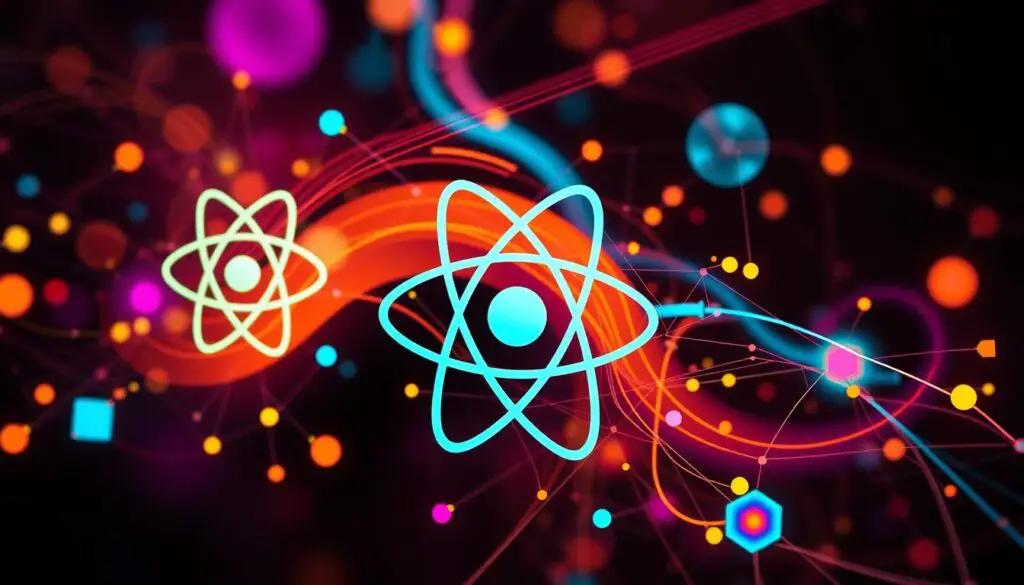
React Native’s functional components make app development easy and efficient. They are defined as simple JavaScript functions. This lets developers focus on making the UI without worrying about state or lifecycle methods.
This way of working fits well with React Native’s core ideas. It lets developers use the same basic ideas they know from web development in mobile apps.
Develop applications using React Native
Core Components: View, Text, and Image
React Native’s core components, like View, Text, and Image, are key for making mobile apps. They are easy to use and make apps look good. This makes it simple to develop applications using React Native for mobile app development and cross-platform development.
The View component is like a div
in web development. It holds other components. Unlike Android, React Native’s View uses Flexbox to arrange components.
The Text component is for showing text in apps. It lets you control font styles and sizes. It’s important for showing information and instructions.
The Image component makes it easy to add images to apps. It supports many formats and lets you adjust size and position. It’s key for making apps look good and feel engaging.
Learning to use these React Native components helps developers make apps that are easy to use. They can make apps that work well on different platforms with React Native.
Props and State Management
Customizing Components with Props
In React Native development, props are key for customizing components. Props, short for “properties,” let you share data between components. This way, you can change how your app looks and works.
Many Core Components in React Native, like View, Text, and Image, can be tweaked with props. Using props, you can adjust things like size, color, and how interactive a component is.
Using State with the useState Hook
To handle changing data in your React Native app, the useState Hook is a great tool. It lets you add state to function components. This makes it easy to manage data that changes or comes from user actions.
The useState Hook sets up a “state variable” with a starting value and a way to change it. This helps update your app’s UI smoothly when data changes. It makes your app feel fast and smooth for users.
State Management Tool | Benefits |
---|---|
Redux | Provides a predictable state container, making it easier to manage complex application state. |
Recoil | Simplifies state management with a lightweight and flexible approach, especially for smaller applications. |
Hooks (useState) | Allows for easy state management in functional components, reducing the need for class components. |
Learning about props and state in React Native helps developers make apps that are both customizable and responsive. These apps offer a great user experience.
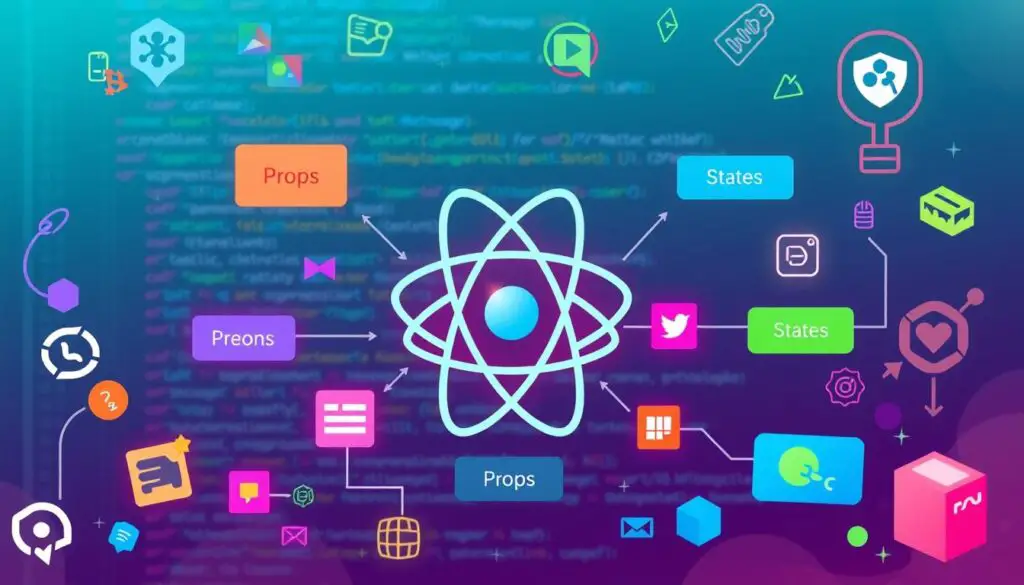
Handling User Interactions
TextInput Component
In React Native, the TextInput component is key for user interactions in mobile apps. It lets users type text, making it easy for them to interact with your cross-platform development projects.
The TextInput component has many customizable options. You can use onChangeText
to catch text changes. The onSubmitEditing
prop helps when the user submits text, opening up more interaction and React Native navigation possibilities.
Using the TextInput component makes your apps more interactive and responsive with React Native. This lets you create engaging mobile app development experiences. These experiences blend user input with your app’s main features and React Native APIs smoothly.
Also, using performance optimization and debugging tools is crucial with the TextInput component. This keeps your apps fast and smooth, even when lots of users are using them. By following React Native best practices, your apps will be enjoyable and user-centric.
Conclusion
In this article, we’ve looked at the basics of developing applications using React Native. This is a well-liked framework for mobile app development. We talked about the importance of React Native components, navigation, and APIs. We also covered how to improve performance optimization, use debugging tools, and handle deployment.
We pointed out the main advantages of React Native. It makes cross-platform development easier. It also speeds up the development process and allows for code reusability. Plus, it offers a native-like performance for users. We also mentioned the strong community support and how easy it is to learn because of JavaScript.
The mobile app industry is booming. It’s expected to hit $673.7 billion by 2027. React Native is a top choice for cross-platform mobile app development. Big names like Facebook, Microsoft Office, Amazon, and Shopify use it. This shows React Native’s value in mobile app development. It’s likely to stay a top pick for developers aiming for efficient, high-quality apps.