How do you implement animations in Flutter?
Flutter is Google’s open-source UI toolkit for making apps for mobile, web, and desktop. It has a strong animation system for creating custom animations. These animations are key to improving user experience and making apps more fun to use.
This guide will show you how to make your app’s UI look amazing with custom animations. Flutter makes it simple to add different animations. Many widgets, like Material widgets, already have animations built-in. But you can also change these to match your app’s style.
Understanding Flutter’s Animation System
Flutter’s animation system uses typed Animation objects. Widgets can either use these animations directly or build more complex ones. This lets them pass animations to other widgets.
Key Concepts and Classes
The core of Flutter’s animation system includes:
- Animation class: Represents a value that changes over time, providing access to the current value and state.
- AnimationController: Controls the playback of an animation, allowing developers to start, stop, and reverse the animation.
- CurvedAnimation: Applies a curve to an animation, enabling non-linear changes in the animation’s value over time.
- Tween: Defines the range of values an animation should interpolate between, such as from 0 to 1 or from one color to another.
These classes help developers create various animations in Flutter apps. By knowing the Animation class, AnimationController, and related concepts, they can make user interfaces that are sophisticated, responsive, and engaging.
Concept | Description | Key Classes |
---|---|---|
Animation State Changes | Tracking the current state of an animation, such as started, paused, or completed. | Animation, AnimationController |
Animation Listeners | Registering callbacks to be notified of changes in an animation’s value or state. | AnimationController, Tween |
Physics-based Animations | Creating animations that mimic real-world dynamics, such as springs, friction, and gravity. | Simulation, SpringSimulation |
By mastering these key concepts and classes, developers can use Flutter’s animation capabilities. This helps them create engaging and responsive user experiences.
Implementing Animated Widgets in Flutter
Flutter is a popular framework for creating apps on multiple platforms. It has a wide range of animated widgets. These widgets make it easy to add animations to your app. You can use them for simple effects like fade and slide, or for more complex animations.
Implicit Animations
The AnimatedContainer is a key animated widget in Flutter. It automatically changes its size, color, or padding. This makes animations easy without needing to manage them yourself. Other widgets like AnimatedSize, AnimatedOpacity, AnimatedSlide, and AnimatedAlign also offer specific animation options.
Explicit Animations
For more control, Flutter has the Transform widget. It lets you scale, rotate, or translate your widgets. You can use Transform.scale, Transform.rotate, and Transform.translate for custom animations. The TweenAnimationBuilder widget is also powerful. It combines Tween, Curve, and Duration for smooth animations.
Animation Management
The heart of Flutter’s animation system is the AnimationController. It controls your animations’ state and progress. You can start, stop, or reverse animations with _controller.forward().
Animated Widget | Description |
---|---|
AnimatedContainer | Automatically animates changes in its properties, such as size, color, or padding. |
AnimatedSize | Animates the size of its child widget, changing it gradually over time. |
AnimatedOpacity | Animates the opacity of its child widget, transitioning between visible and invisible states. |
Transform | Applies transformations like scaling, rotation, and translation to its child widgets. |
TweenAnimationBuilder | Combines Tween, Curve, and Duration to create smooth and interactive animations. |
Flutter’s animated widgets and tools help you make your app more engaging. You can create dynamic and visually appealing user experiences. This improves user satisfaction and keeps them interested in your app.
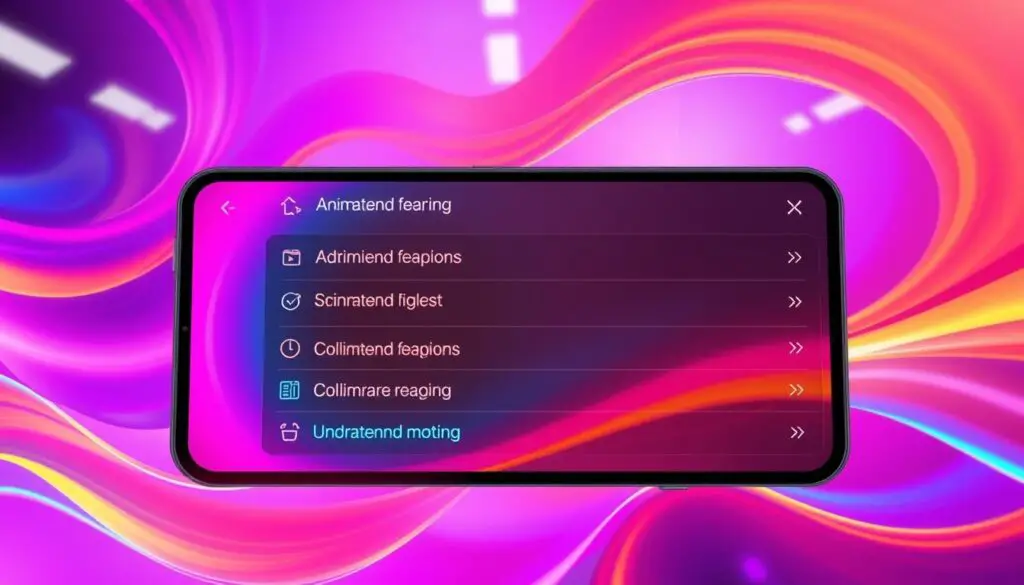
Creating Custom Animations with AnimatedBuilder
Flutter’s pre-built animated widgets are great, but sometimes you need something special. That’s where AnimatedBuilder and AnimatedWidget come in. They let you make your own animations from scratch.
Building Explicit Animations
The AnimatedBuilder widget is key for making custom animations. You can mix Animation, Tween, and AnimationController to control your animations. This means you can design exactly how you want your UI/UX to look and feel.
For instance, to make an opacity animation, use AnimatedBuilder with an AnimationController and a Tween. The AnimationController handles the animation’s life cycle. The Tween sets the animation’s start and end points. Then, AnimatedBuilder makes the widget’s opacity change smoothly.
You can also change a widget’s position, size, or rotation using the Transform widget. By mixing different animations and trying out different curves, you can make complex animations. These can make your app more engaging and beautiful.
Learning the basics of animation and using Flutter’s tools is crucial. It helps you make animations that are both smooth and visually appealing. This skill is key for creating interactive and engaging user experiences in your apps.
Animation Property | Value |
---|---|
Duration | 1 second |
Initial Size | 160 – 180 |
Animation Trigger | Start: `_animationController.forward()` Stop: `_animationController.stop()` |
Resource Management | Dispose `_animationController.dispose()` |
App development using Flutter
Flutter is Google’s open-source UI toolkit for making top-notch mobile apps for iOS and Android. It uses Dart, a programming language, for fast and easy app development. Flutter’s reactive model and wide range of widgets make it great for building apps for different platforms.
Flutter stands out because it offers a smooth user experience on both iOS and Android. Developers can write one codebase for apps that look and feel native on both platforms. This makes app development faster and ensures a great user experience.
Flutter’s performance is impressive. It uses Skia, a graphics engine, to run smooth animations on even low-end devices. Dart’s efficiency helps Flutter deliver fast and smooth app performance.
Flutter also has a vast widget ecosystem. It offers everything from basic UI elements to complex interactive components. This flexibility lets developers build stunning and responsive apps.
Flutter works well with Google’s services, like Firebase. This integration makes app development easier by handling tasks like authentication and database management. It’s a one-stop solution for many app development needs.
As the app market grows, Flutter is becoming a top choice for app development. Its ability to make app development easier, deliver high-performance apps, and offer a wide range of widgets and services is attractive to businesses and developers.
Framework | Language | Community | Performance | UI Customization |
---|---|---|---|---|
Flutter | Dart | Growing | Excellent | Highly Customizable |
React Native | JavaScript | Established | Good | Customizable |
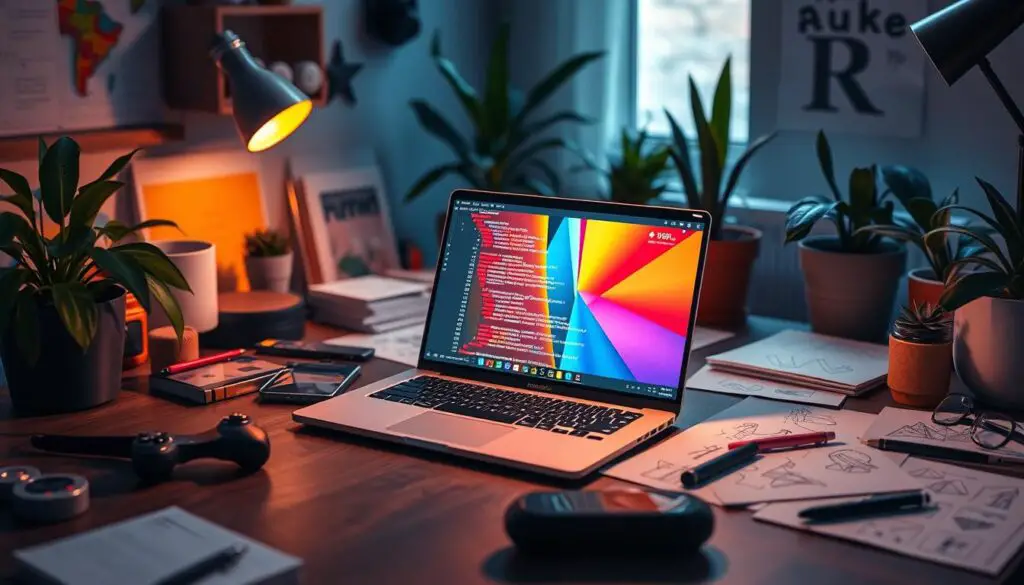
In conclusion, Flutter is a top choice for app development. Its versatility, performance, and community support make it ideal. Developers can create high-quality apps that engage users and help businesses succeed.
Best Practices for Optimizing Flutter Animations
Performance Considerations
Smooth and efficient animations are key for a great user experience in Flutter apps. Flutter’s animation system is powerful, but following best practices is crucial. This ensures your app runs smoothly and looks great.
- Leveraging const Constructors: Use const constructors on widgets to improve performance. It helps Flutter do less work during rebuilds.
- Implementing Lazy Building: Use lazy builder methods for big grids or lists. This way, only what’s on screen is built at startup.
- Avoiding Expensive Operations: Avoid too many calls to
saveLayer()
to prevent jank. Also, use opacity and clipping wisely for better performance. - Optimizing Intrinsic Passes: Intrinsic passes can slow down your app by causing extra layout passes. They’re needed for grids and lists to size cells evenly.
- Achieving Frame Rate Targets: Aim to build and display frames in 16ms for a smooth 60 FPS experience. This is key for good performance.
Frame rate is vital for a smooth user experience. A higher FPS means better performance. The complexity of your widget tree, state management, and UI elements also affect performance.
Use tools like Flutter DevTools for real-time performance monitoring. It tracks FPS, build times, and the rendering pipeline. The Dart observatory helps with memory issues, showing memory usage and allocation.
By following these best practices and using performance optimization techniques, Flutter developers can make their apps run smoothly. This is true even on devices with less power or resources.
Conclusion
In this guide, we’ve looked at how to add animations to Flutter. Flutter is a powerful tool for making mobile apps look great and work fast. We covered the basics of Flutter’s animation system and how to use pre-built widgets.
We also learned about creating custom animations with AnimatedBuilder and AnimatedWidget. This makes apps more engaging and fun to use.
Flutter is known for its fast development and high performance. It’s free and open-source, making it accessible to many developers. This has made Flutter a favorite among developers worldwide.
Now, as we wrap up, it’s clear Flutter is a big hit in the app development world. It’s used by 46% of developers globally. Its inclusion in the top 10 frameworks shows its growing popularity.
While learning Dart, Flutter’s programming language, can be tough, its future looks bright. This makes Flutter a great choice for creating modern, eye-catching mobile apps.