How do you handle background tasks in Android using Kotlin?
Android apps often need to do tasks in the background, like making network calls or reading from databases. These tasks should run smoothly to keep the app working well and feel fast to users. We’ll look at how to handle these tasks well using Kotlin.
Android developers have many ways to manage background tasks. Each method has its own benefits and drawbacks. The right choice depends on the task’s length, if it needs to keep running in the background, and how it affects the user. In this guide, we’ll cover the best ways to manage background tasks in Kotlin-based Android apps.
Understanding Background Tasks in Android
In Android App Development Using Kotlin, it’s key to handle background tasks well. This keeps the app running smoothly. The main thread handles the UI and user actions but isn’t for long tasks. Doing so can cause the app to crash or freeze.
Common Examples of Background Tasks
Some common background tasks in Android include:
- Making network requests, such as fetching data from an API
- Performing I/O operations, like reading or writing to a database
- Executing heavy read/write operations, such as processing large amounts of data
Importance of Handling Background Tasks Efficiently
Handling these Background Tasks well is vital for a smooth app. The main thread must update the screen every 16ms to keep a smooth flow. If it’s blocked, the app becomes slow, ruining the user’s experience.
By moving these tasks to other threads or using Callback-Style Functions, developers keep the main thread free. This makes the app more responsive and fun to use in Android App Development Using Kotlin.
Android App Development Using Kotlin
The Kotlin programming language is now a top pick for Android app development. It was introduced by JetBrains in 2011 and became an official language for Android development by Google in 2017. Kotlin is known for being modern, concise, and easy to use with the Android platform.
Kotlin has many features that make it great for Android applications. It works well with Java, making it easy for developers to mix code. This leads to better code quality, faster development, and lower costs.
One big plus of Kotlin is its ability to prevent common errors like null pointer exceptions. This makes the code safer and helps developers work more efficiently.
Kotlin also makes background task management easier with coroutines. This means developers can handle tasks more smoothly. Plus, Kotlin’s expressive and concise syntax lets developers do more with less code.
Kotlin is also great because it works well with Java and supports many platforms. This includes JavaScript, Android, Linux, Mac, and Windows. Big names like Uber, Pinterest, Trello, and Twitter use Kotlin, showing its reliability.
As Google keeps improving Kotlin, its future in Android app development looks bright. With its concise syntax, null safety, and improved productivity, Kotlin is a top choice for making high-quality Android apps.
Coroutines: The Recommended Solution
In Android app development with Kotlin, Kotlin coroutines are a top choice for background tasks. They make asynchronous code easier to read and manage. This makes the code more structured and simpler to keep up with.
Introduction to Coroutines and Suspending Functions
Coroutines in Kotlin use suspending functions to pause and resume without blocking the main thread. This lets developers write code that looks like it’s running one step at a time. It’s perfect for tasks like database or network access that shouldn’t slow down the app.
Coroutine Dispatchers: Managing Thread Execution
Coroutine dispatchers like Dispatchers.Main
, Dispatchers.Default
, and Dispatchers.IO
help manage threads. Developers can switch between the main thread and background threads as needed. This ensures the app runs smoothly and efficiently.
More than 50% of Android developers say coroutines have boosted their productivity. Kotlin 1.3 made async code easier, and Jetpack libraries now support coroutines fully. This leads to better performance and fewer memory leaks.
Choosing the right dispatcher is key when creating coroutines in Android apps. For example, Dispatchers.IO
is great for I/O operations. It keeps the app running smoothly. The ViewModel
Architecture also works well with coroutines for background tasks.
Alternatives for Background Task Management
While Kotlin coroutines are the top choice for Android App Development Using Kotlin, other options exist. Asynchronous work options like AsyncTask and HandlerThread are good for short tasks. They help talk to the UI thread.
Task scheduling APIs such as WorkManager and JobScheduler are better for long tasks. They keep tasks running even when the app is closed.
Asynchronous Work Options
AsyncTask is a common tool for simple tasks. But, Kotlin coroutines are now the better choice. They make handling tasks easier and cleaner.
Task Scheduling APIs
WorkManager and JobScheduler are great for complex tasks. They make sure tasks get done, even when the app is not open.
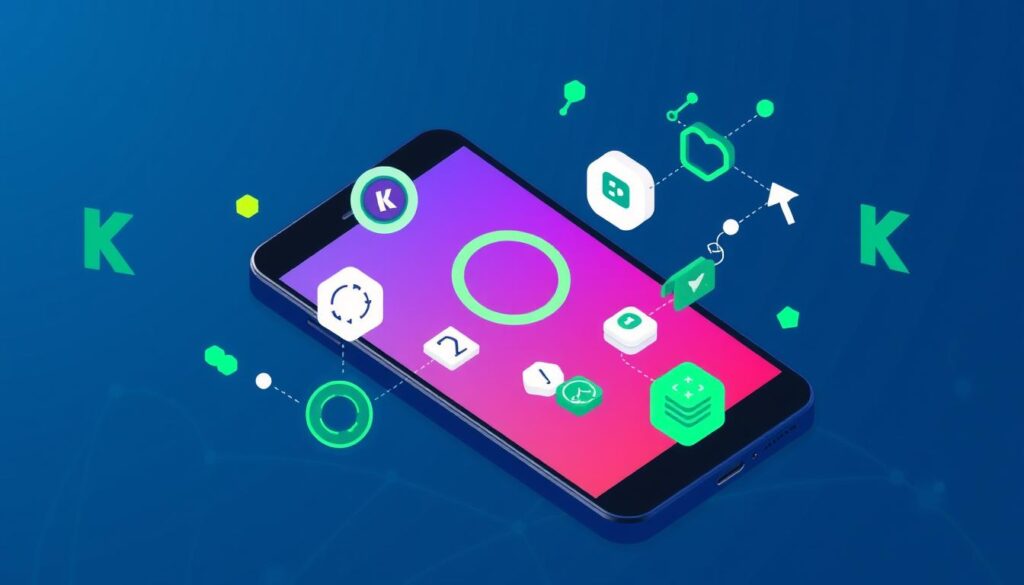
Knowing when to use each option is key. It helps make Android App Development Using Kotlin apps better and faster.
Foreground Services: When to Use and Alternatives
In Android app development with Kotlin, foreground services are key for tasks that can’t be paused. Yet, they have limits and might affect app performance and security. It’s crucial to know when to use them and what else is available.
Android has strict rules for foreground services. Starting with Android 13, users can easily dismiss these services’ notifications. Also, Android 12 and later wait 10 seconds before showing a notification for short services.
For certain tasks, there are better options than foreground services. For example, media playback can use picture-in-picture mode. For Bluetooth tasks, the companion device manager is a good alternative.
Foreground Service Types and Requirements
Android 14 introduced new service types like health, remoteMessaging, shortService, specialUse, and systemExempted. Each type has its own rules and permissions for developers.
- For API level 29 or higher, services using location must declare it.
- For API level 30 or higher, camera or microphone services need a declaration.
- From API level 34, all services must declare their type.
Developers should only use the service types they really need. This meets system standards and avoids problems.
Foreground Service Type | Requirements |
---|---|
dataSync | Permissions for network or Bluetooth connections |
health | Permissions related to body sensors or activity recognition |
location | Enabled location services and user-granted runtime permissions for location access |
Knowing when to use foreground services and what else is out there helps Kotlin developers make better apps. These apps will meet system standards and give users a smooth experience.
Comparing Background Task Handling Methods
As an Android developer using Kotlin, you have many options for managing background tasks. Each method has its own strengths and weaknesses. We’ll look at different techniques like AsyncTask, HandlerThread, and more. This includes JobScheduler, WorkManager, and Kotlin Coroutines.
AsyncTask and HandlerThread
AsyncTask is a common choice for background tasks. It makes it easy to run tasks on a separate thread and update the UI. But, it can’t check if the parent activity still exists, which might cause problems. HandlerThread gives you more control over background threads.
IntentService and JobScheduler
IntentService is great for background tasks. It handles thread management and stops the task before stopping the service. JobScheduler is newer and lets you schedule tasks based on device conditions like network and battery.
WorkManager and Executors
WorkManager makes managing background tasks easier. It offers different task types for your app’s needs. Executors let you manage your own thread pools for tasks.
RxJava and Android Services
RxJava is a top choice for handling asynchronous tasks. It’s great for complex task chains and error handling. Android Services are also good for long tasks, with options for foreground and background.
Kotlin Coroutines
Kotlin’s Coroutines are now the top pick for background tasks. They are lightweight and offer better lifecycle control. They work well with Kotlin’s features, making code cleaner and easier to maintain.
Knowing the pros and cons of each method helps you choose the best for your Android app. This ensures efficient and reliable background task management with Kotlin.
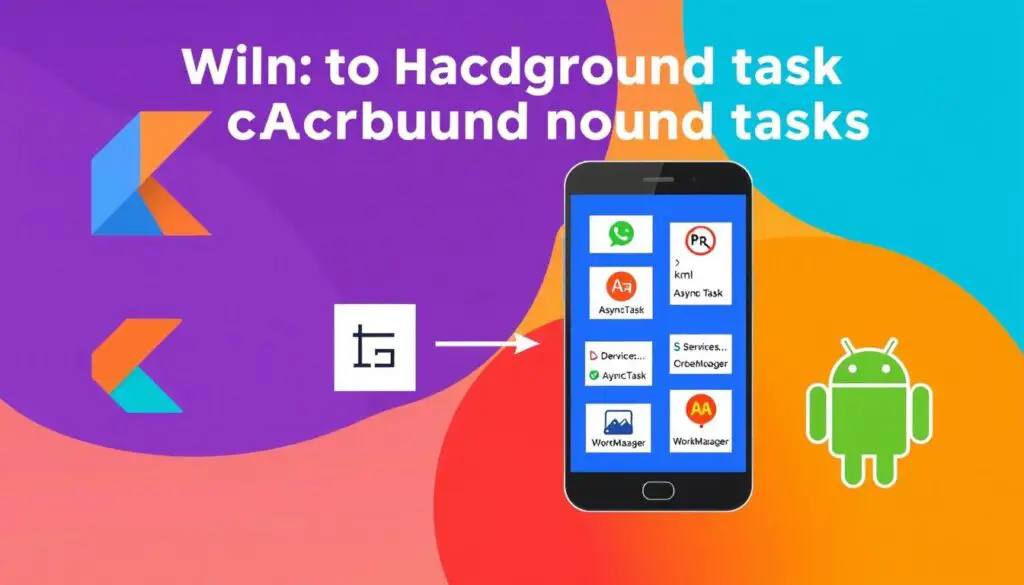
Conclusion
In this article, we looked at how to handle background tasks in Android app development with Kotlin. We saw why it’s key to manage tasks well for a smooth app experience. We also learned about Kotlin coroutines, the top choice for Background Task Management.
Kotlin coroutines make handling tasks easier with suspending functions and dispatchers. We also talked about other ways, like Asynchronous Work and Task Scheduling APIs. Foreground services were discussed too, with their pros and cons.
This knowledge helps you pick the best method for your Android apps. You’ll make your apps more enjoyable for users. We highlighted Kotlin Coroutines as the best for async programming in Kotlin.
Coroutines make coding for background tasks simpler and more efficient. We also compared different methods for handling tasks. This gives you a full view of what’s available and when to use them.